Parts¶
The following classes allow interaction with a vessels individual parts.
Parts¶
- class Parts¶
Instances of this class are used to interact with the parts of a vessel. An instance can be obtained by calling
Vessel.Parts
.- Part Root { get; }¶
The vessels root part.
- Game Scenes:
All
Note
See the discussion on Trees of Parts.
- IList<Part> WithName (String name)¶
A list of parts whose
Part.Name
is name.- Parameters:
- Game Scenes:
All
- IList<Part> WithTitle (String title)¶
A list of all parts whose
Part.Title
is title.- Parameters:
- Game Scenes:
All
- IList<Part> WithTag (String tag)¶
A list of all parts whose
Part.Tag
is tag.- Parameters:
- Game Scenes:
All
- IList<Part> WithModule (String moduleName)¶
A list of all parts that contain a
Module
whoseModule.Name
is moduleName.- Parameters:
- Game Scenes:
All
- IList<Part> InStage (Int32 stage)¶
A list of all parts that are activated in the given stage.
- Parameters:
- Game Scenes:
All
Note
See the discussion on Staging.
- IList<Part> InDecoupleStage (Int32 stage)¶
A list of all parts that are decoupled in the given stage.
- Parameters:
- Game Scenes:
All
Note
See the discussion on Staging.
- IList<Module> ModulesWithName (String moduleName)¶
A list of modules (combined across all parts in the vessel) whose
Module.Name
is moduleName.- Parameters:
- Game Scenes:
All
- IList<Antenna> Antennas { get; }¶
A list of all antennas in the vessel.
- Game Scenes:
All
Note
If RemoteTech is installed, this will always return an empty list. To interact with RemoteTech antennas, use the RemoteTech service APIs.
- IList<ControlSurface> ControlSurfaces { get; }¶
A list of all control surfaces in the vessel.
- Game Scenes:
All
- IList<DockingPort> DockingPorts { get; }¶
A list of all docking ports in the vessel.
- Game Scenes:
All
- IList<Engine> Engines { get; }¶
A list of all engines in the vessel.
- Game Scenes:
All
Note
This includes any part that generates thrust. This covers many different types of engine, including liquid fuel rockets, solid rocket boosters, jet engines and RCS thrusters.
- IList<Experiment> Experiments { get; }¶
A list of all science experiments in the vessel.
- Game Scenes:
All
- IList<LaunchClamp> LaunchClamps { get; }¶
A list of all launch clamps attached to the vessel.
- Game Scenes:
All
- IList<ResourceDrain> ResourceDrains { get; }¶
A list of all resource drains in the vessel.
- Game Scenes:
All
- IList<ReactionWheel> ReactionWheels { get; }¶
A list of all reaction wheels in the vessel.
- Game Scenes:
All
- IList<ResourceConverter> ResourceConverters { get; }¶
A list of all resource converters in the vessel.
- Game Scenes:
All
- IList<ResourceHarvester> ResourceHarvesters { get; }¶
A list of all resource harvesters in the vessel.
- Game Scenes:
All
- IList<RoboticHinge> RoboticHinges { get; }¶
A list of all robotic hinges in the vessel.
- Game Scenes:
All
- IList<RoboticPiston> RoboticPistons { get; }¶
A list of all robotic pistons in the vessel.
- Game Scenes:
All
- IList<RoboticRotation> RoboticRotations { get; }¶
A list of all robotic rotations in the vessel.
- Game Scenes:
All
- IList<RoboticRotor> RoboticRotors { get; }¶
A list of all robotic rotors in the vessel.
- Game Scenes:
All
- IList<SolarPanel> SolarPanels { get; }¶
A list of all solar panels in the vessel.
- Game Scenes:
All
Part¶
- class Part¶
Represents an individual part. Vessels are made up of multiple parts. Instances of this class can be obtained by several methods in
Parts
.- String Name { get; }¶
Internal name of the part, as used in part cfg files. For example “Mark1-2Pod”.
- Game Scenes:
All
- String Title { get; }¶
Title of the part, as shown when the part is right clicked in-game. For example “Mk1-2 Command Pod”.
- Game Scenes:
All
- String Tag { get; set; }¶
The name tag for the part. Can be set to a custom string using the in-game user interface.
- Game Scenes:
All
Note
This string is shared with kOS if it is installed.
- Tuple<Double, Double, Double> HighlightColor { get; set; }¶
The color used to highlight the part, as an RGB triple.
- Game Scenes:
All
- Part Parent { get; }¶
The parts parent. Returns
null
if the part does not have a parent. This, in combination withPart.Children
, can be used to traverse the vessels parts tree.- Game Scenes:
All
Note
See the discussion on Trees of Parts.
- IList<Part> Children { get; }¶
The parts children. Returns an empty list if the part has no children. This, in combination with
Part.Parent
, can be used to traverse the vessels parts tree.- Game Scenes:
All
Note
See the discussion on Trees of Parts.
- Boolean AxiallyAttached { get; }¶
Whether the part is axially attached to its parent, i.e. on the top or bottom of its parent. If the part has no parent, returns
false
.- Game Scenes:
All
Note
See the discussion on Attachment Modes.
- Boolean RadiallyAttached { get; }¶
Whether the part is radially attached to its parent, i.e. on the side of its parent. If the part has no parent, returns
false
.- Game Scenes:
All
Note
See the discussion on Attachment Modes.
- Int32 Stage { get; }¶
The stage in which this part will be activated. Returns -1 if the part is not activated by staging.
- Game Scenes:
All
Note
See the discussion on Staging.
- Int32 DecoupleStage { get; }¶
The stage in which this part will be decoupled. Returns -1 if the part is never decoupled from the vessel.
- Game Scenes:
All
Note
See the discussion on Staging.
- Double Mass { get; }¶
The current mass of the part, including resources it contains, in kilograms. Returns zero if the part is massless.
- Game Scenes:
All
- Double DryMass { get; }¶
The mass of the part, not including any resources it contains, in kilograms. Returns zero if the part is massless.
- Game Scenes:
All
- Boolean Shielded { get; }¶
Whether the part is shielded from the exterior of the vessel, for example by a fairing.
- Game Scenes:
All
- Single DynamicPressure { get; }¶
The dynamic pressure acting on the part, in Pascals.
- Game Scenes:
All
- Double ImpactTolerance { get; }¶
The impact tolerance of the part, in meters per second.
- Game Scenes:
All
- Double MaxTemperature { get; }¶
Maximum temperature that the part can survive, in Kelvin.
- Game Scenes:
All
- Double MaxSkinTemperature { get; }¶
Maximum temperature that the skin of the part can survive, in Kelvin.
- Game Scenes:
All
- Single ThermalMass { get; }¶
A measure of how much energy it takes to increase the internal temperature of the part, in Joules per Kelvin.
- Game Scenes:
All
- Single ThermalSkinMass { get; }¶
A measure of how much energy it takes to increase the skin temperature of the part, in Joules per Kelvin.
- Game Scenes:
All
- Single ThermalResourceMass { get; }¶
A measure of how much energy it takes to increase the temperature of the resources contained in the part, in Joules per Kelvin.
- Game Scenes:
All
- Single ThermalConductionFlux { get; }¶
The rate at which heat energy is conducting into or out of the part via contact with other parts. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Game Scenes:
All
- Single ThermalConvectionFlux { get; }¶
The rate at which heat energy is convecting into or out of the part from the surrounding atmosphere. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Game Scenes:
All
- Single ThermalRadiationFlux { get; }¶
The rate at which heat energy is radiating into or out of the part from the surrounding environment. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Game Scenes:
All
- Single ThermalInternalFlux { get; }¶
The rate at which heat energy is begin generated by the part. For example, some engines generate heat by combusting fuel. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Game Scenes:
All
- Single ThermalSkinToInternalFlux { get; }¶
The rate at which heat energy is transferring between the part’s skin and its internals. Measured in energy per unit time, or power, in Watts. A positive value means the part’s internals are gaining heat energy, and negative means its skin is gaining heat energy.
- Game Scenes:
All
- IList<Part> FuelLinesFrom { get; }¶
The parts that are connected to this part via fuel lines, where the direction of the fuel line is into this part.
- Game Scenes:
All
Note
See the discussion on Fuel Lines.
- IList<Part> FuelLinesTo { get; }¶
The parts that are connected to this part via fuel lines, where the direction of the fuel line is out of this part.
- Game Scenes:
All
Note
See the discussion on Fuel Lines.
- Antenna Antenna { get; }¶
An
Antenna
if the part is an antenna, otherwisenull
.- Game Scenes:
All
Note
If RemoteTech is installed, this will always return
null
. To interact with RemoteTech antennas, use the RemoteTech service APIs.
- ControlSurface ControlSurface { get; }¶
A
ControlSurface
if the part is an aerodynamic control surface, otherwisenull
.- Game Scenes:
All
- Decoupler Decoupler { get; }¶
A
Decoupler
if the part is a decoupler, otherwisenull
.- Game Scenes:
All
- DockingPort DockingPort { get; }¶
A
DockingPort
if the part is a docking port, otherwisenull
.- Game Scenes:
All
- Experiment Experiment { get; }¶
An
Experiment
if the part contains a single science experiment, otherwisenull
.- Game Scenes:
All
Note
Throws an exception if the part contains more than one experiment. In that case, use
Part.Experiments
to get the list of experiments in the part.
- IList<Experiment> Experiments { get; }¶
A list of
Experiment
objects that the part contains.- Game Scenes:
All
- Intake Intake { get; }¶
An
Intake
if the part is an intake, otherwisenull
.- Game Scenes:
All
Note
This includes any part that generates thrust. This covers many different types of engine, including liquid fuel rockets, solid rocket boosters and jet engines. For RCS thrusters see
RCS
.
- LaunchClamp LaunchClamp { get; }¶
A
LaunchClamp
if the part is a launch clamp, otherwisenull
.- Game Scenes:
All
- Parachute Parachute { get; }¶
A
Parachute
if the part is a parachute, otherwisenull
.- Game Scenes:
All
- ResourceDrain ResourceDrain { get; }¶
A
ResourceDrain
if the part is a resource drain, otherwisenull
.- Game Scenes:
All
- ReactionWheel ReactionWheel { get; }¶
A
ReactionWheel
if the part is a reaction wheel, otherwisenull
.- Game Scenes:
All
- ResourceConverter ResourceConverter { get; }¶
A
ResourceConverter
if the part is a resource converter, otherwisenull
.- Game Scenes:
All
- ResourceHarvester ResourceHarvester { get; }¶
A
ResourceHarvester
if the part is a resource harvester, otherwisenull
.- Game Scenes:
All
- RoboticController RoboticController { get; }¶
A
RoboticController
if the part is a robotic controller, otherwisenull
.- Game Scenes:
All
- RoboticHinge RoboticHinge { get; }¶
A
RoboticHinge
if the part is a robotic hinge, otherwisenull
.- Game Scenes:
All
- RoboticPiston RoboticPiston { get; }¶
A
RoboticPiston
if the part is a robotic piston, otherwisenull
.- Game Scenes:
All
- RoboticRotation RoboticRotation { get; }¶
A
RoboticRotation
if the part is a robotic rotation servo, otherwisenull
.- Game Scenes:
All
- RoboticRotor RoboticRotor { get; }¶
A
RoboticRotor
if the part is a robotic rotor, otherwisenull
.- Game Scenes:
All
- SolarPanel SolarPanel { get; }¶
A
SolarPanel
if the part is a solar panel, otherwisenull
.- Game Scenes:
All
- Tuple<Double, Double, Double> Position (ReferenceFrame referenceFrame)¶
The position of the part in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned position vector is in.
- Returns:
The position as a vector.
- Game Scenes:
All
Note
This is a fixed position in the part, defined by the parts model. It s not necessarily the same as the parts center of mass. Use
Part.CenterOfMass
to get the parts center of mass.
- Tuple<Double, Double, Double> CenterOfMass (ReferenceFrame referenceFrame)¶
The position of the parts center of mass in the given reference frame. If the part is physicsless, this is equivalent to
Part.Position
.- Parameters:
referenceFrame – The reference frame that the returned position vector is in.
- Returns:
The position as a vector.
- Game Scenes:
All
- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> BoundingBox (ReferenceFrame referenceFrame)¶
The axis-aligned bounding box of the part in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned position vectors are in.
- Returns:
The positions of the minimum and maximum vertices of the box, as position vectors.
- Game Scenes:
All
Note
This is computed from the collision mesh of the part. If the part is not collidable, the box has zero volume and is centered on the
Part.Position
of the part.
- Tuple<Double, Double, Double> Direction (ReferenceFrame referenceFrame)¶
The direction the part points in, in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned direction is in.
- Returns:
The direction as a unit vector.
- Game Scenes:
All
- Tuple<Double, Double, Double> Velocity (ReferenceFrame referenceFrame)¶
The linear velocity of the part in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned velocity vector is in.
- Returns:
The velocity as a vector. The vector points in the direction of travel, and its magnitude is the speed of the body in meters per second.
- Game Scenes:
All
- Tuple<Double, Double, Double, Double> Rotation (ReferenceFrame referenceFrame)¶
The rotation of the part, in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned rotation is in.
- Returns:
The rotation as a quaternion of the form \((x, y, z, w)\).
- Game Scenes:
All
- Tuple<Double, Double, Double> MomentOfInertia { get; }¶
The moment of inertia of the part in \(kg.m^2\) around its center of mass in the parts reference frame (
ReferenceFrame
).- Game Scenes:
All
- IList<Double> InertiaTensor { get; }¶
The inertia tensor of the part in the parts reference frame (
ReferenceFrame
). Returns the 3x3 matrix as a list of elements, in row-major order.- Game Scenes:
All
- ReferenceFrame ReferenceFrame { get; }¶
The reference frame that is fixed relative to this part, and centered on a fixed position within the part, defined by the parts model.
The origin is at the position of the part, as returned by
Part.Position
.The axes rotate with the part.
The x, y and z axis directions depend on the design of the part.
- Game Scenes:
All
Note
For docking port parts, this reference frame is not necessarily equivalent to the reference frame for the docking port, returned by
DockingPort.ReferenceFrame
.Mk1 Command Pod reference frame origin and axes¶
- ReferenceFrame CenterOfMassReferenceFrame { get; }¶
The reference frame that is fixed relative to this part, and centered on its center of mass.
The origin is at the center of mass of the part, as returned by
Part.CenterOfMass
.The axes rotate with the part.
The x, y and z axis directions depend on the design of the part.
- Game Scenes:
All
Note
For docking port parts, this reference frame is not necessarily equivalent to the reference frame for the docking port, returned by
DockingPort.ReferenceFrame
.
- Force AddForce (Tuple<Double, Double, Double> force, Tuple<Double, Double, Double> position, ReferenceFrame referenceFrame)¶
Exert a constant force on the part, acting at the given position.
- Parameters:
force – A vector pointing in the direction that the force acts, with its magnitude equal to the strength of the force in Newtons.
position – The position at which the force acts, as a vector.
referenceFrame – The reference frame that the force and position are in.
- Returns:
An object that can be used to remove or modify the force.
- Game Scenes:
All
- void InstantaneousForce (Tuple<Double, Double, Double> force, Tuple<Double, Double, Double> position, ReferenceFrame referenceFrame)¶
Exert an instantaneous force on the part, acting at the given position.
- Parameters:
force – A vector pointing in the direction that the force acts, with its magnitude equal to the strength of the force in Newtons.
position – The position at which the force acts, as a vector.
referenceFrame – The reference frame that the force and position are in.
- Game Scenes:
All
Note
The force is applied instantaneously in a single physics update.
- AutoStrutMode AutoStrutMode { get; }¶
Auto-strut mode.
- Game Scenes:
All
- enum AutoStrutMode¶
The state of an auto-strut.
Part.AutoStrutMode
- Off¶
Off
- Root¶
Root
- Heaviest¶
Heaviest
- Grandparent¶
Grandparent
- ForceRoot¶
ForceRoot
- ForceHeaviest¶
ForceHeaviest
- ForceGrandparent¶
ForceGrandparent
- class Force¶
Obtained by calling
Part.AddForce
.- Tuple<Double, Double, Double> ForceVector { get; set; }¶
The force vector, in Newtons.
- Returns:
A vector pointing in the direction that the force acts, with its magnitude equal to the strength of the force in Newtons.
- Game Scenes:
All
- Tuple<Double, Double, Double> Position { get; set; }¶
The position at which the force acts, in reference frame
ReferenceFrame
.- Returns:
The position as a vector.
- Game Scenes:
All
- ReferenceFrame ReferenceFrame { get; set; }¶
The reference frame of the force vector and position.
- Game Scenes:
All
- void Remove ()¶
Remove the force.
- Game Scenes:
All
Module¶
- class Module¶
This can be used to interact with a specific part module. This includes part modules in stock KSP, and those added by mods.
In KSP, each part has zero or more PartModules associated with it. Each one contains some of the functionality of the part. For example, an engine has a “ModuleEngines” part module that contains all the functionality of an engine.
- IDictionary<String, String> Fields { get; }¶
The modules field names and their associated values, as a dictionary. These are the values visible in the right-click menu of the part.
- Game Scenes:
All
Note
Throws an exception if there is more than one field with the same name. In that case, use
Module.FieldsById
to get the fields by identifier.
- IDictionary<String, String> FieldsById { get; }¶
The modules field identifiers and their associated values, as a dictionary. These are the values visible in the right-click menu of the part.
- Game Scenes:
All
- Boolean HasField (String name)¶
Returns
true
if the module has a field with the given name.- Parameters:
name – Name of the field.
- Game Scenes:
All
- Boolean HasFieldWithId (String id)¶
Returns
true
if the module has a field with the given identifier.- Parameters:
id – Identifier of the field.
- Game Scenes:
All
- String GetField (String name)¶
Returns the value of a field with the given name.
- Parameters:
name – Name of the field.
- Game Scenes:
All
- String GetFieldById (String id)¶
Returns the value of a field with the given identifier.
- Parameters:
id – Identifier of the field.
- Game Scenes:
All
- void SetFieldInt (String name, Int32 value)¶
Set the value of a field to the given integer number.
- Parameters:
name – Name of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldIntById (String id, Int32 value)¶
Set the value of a field to the given integer number.
- Parameters:
id – Identifier of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldFloat (String name, Single value)¶
Set the value of a field to the given floating point number.
- Parameters:
name – Name of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldFloatById (String id, Single value)¶
Set the value of a field to the given floating point number.
- Parameters:
id – Identifier of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldString (String name, String value)¶
Set the value of a field to the given string.
- Parameters:
name – Name of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldStringById (String id, String value)¶
Set the value of a field to the given string.
- Parameters:
id – Identifier of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldBool (String name, Boolean value)¶
Set the value of a field to true or false.
- Parameters:
name – Name of the field.
value – Value to set.
- Game Scenes:
All
- void SetFieldBoolById (String id, Boolean value)¶
Set the value of a field to true or false.
- Parameters:
id – Identifier of the field.
value – Value to set.
- Game Scenes:
All
- void ResetField (String name)¶
Set the value of a field to its original value.
- Parameters:
name – Name of the field.
- Game Scenes:
All
- void ResetFieldById (String id)¶
Set the value of a field to its original value.
- Parameters:
id – Identifier of the field.
- Game Scenes:
All
- IList<String> Events { get; }¶
A list of the names of all of the modules events. Events are the clickable buttons visible in the right-click menu of the part.
- Game Scenes:
All
- IList<String> EventsById { get; }¶
A list of the identifiers of all of the modules events. Events are the clickable buttons visible in the right-click menu of the part.
- Game Scenes:
All
- Boolean HasEvent (String name)¶
true
if the module has an event with the given name.- Parameters:
- Game Scenes:
All
- Boolean HasEventWithId (String id)¶
true
if the module has an event with the given identifier.- Parameters:
- Game Scenes:
All
- void TriggerEvent (String name)¶
Trigger the named event. Equivalent to clicking the button in the right-click menu of the part.
- Parameters:
- Game Scenes:
All
- void TriggerEventById (String id)¶
Trigger the event with the given identifier. Equivalent to clicking the button in the right-click menu of the part.
- Parameters:
- Game Scenes:
All
- IList<String> Actions { get; }¶
A list of all the names of the modules actions. These are the parts actions that can be assigned to action groups in the in-game editor.
- Game Scenes:
All
- IList<String> ActionsById { get; }¶
A list of all the identifiers of the modules actions. These are the parts actions that can be assigned to action groups in the in-game editor.
- Game Scenes:
All
- Boolean HasAction (String name)¶
true
if the part has an action with the given name.- Parameters:
- Game Scenes:
All
- Boolean HasActionWithId (String id)¶
true
if the part has an action with the given identifier.- Parameters:
- Game Scenes:
All
Specific Types of Part¶
The following classes provide functionality for specific types of part.
Antenna¶
Note
If RemoteTech is installed, use the RemoteTech service APIs to interact with antennas. This class is only for stock KSP antennas.
- class Antenna¶
An antenna. Obtained by calling
Part.Antenna
.- AntennaState State { get; }¶
The current state of the antenna.
- Game Scenes:
All
- Boolean Deployed { get; set; }¶
Whether the antenna is deployed.
- Game Scenes:
All
Note
Fixed antennas are always deployed. Returns an error if you try to deploy a fixed antenna.
- void Transmit ()¶
Transmit data.
- Game Scenes:
All
- void Cancel ()¶
Cancel current transmission of data.
- Game Scenes:
All
- Boolean AllowPartial { get; set; }¶
Whether partial data transmission is permitted.
- Game Scenes:
All
- Boolean Combinable { get; }¶
Whether the antenna can be combined with other antennae on the vessel to boost the power.
- Game Scenes:
All
Cargo Bay¶
- class CargoBay¶
A cargo bay. Obtained by calling
Part.CargoBay
.- CargoBayState State { get; }¶
The state of the cargo bay.
- Game Scenes:
All
Control Surface¶
- class ControlSurface¶
An aerodynamic control surface. Obtained by calling
Part.ControlSurface
.- Boolean PitchEnabled { get; set; }¶
Whether the control surface has pitch control enabled.
- Game Scenes:
All
- Boolean YawEnabled { get; set; }¶
Whether the control surface has yaw control enabled.
- Game Scenes:
All
- Boolean RollEnabled { get; set; }¶
Whether the control surface has roll control enabled.
- Game Scenes:
All
- Single AuthorityLimiter { get; set; }¶
The authority limiter for the control surface, which controls how far the control surface will move.
- Game Scenes:
All
- Boolean Deployed { get; set; }¶
Whether the control surface has been fully deployed.
- Game Scenes:
All
- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> AvailableTorque { get; }¶
The available torque, in Newton meters, that can be produced by this control surface, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
Vessel.ReferenceFrame
.- Game Scenes:
All
Decoupler¶
- class Decoupler¶
A decoupler. Obtained by calling
Part.Decoupler
- Vessel Decouple ()¶
Fires the decoupler. Returns the new vessel created when the decoupler fires. Throws an exception if the decoupler has already fired.
- Game Scenes:
All
Note
When called, the active vessel may change. It is therefore possible that, after calling this function, the object(s) returned by previous call(s) to
SpaceCenter.ActiveVessel
no longer refer to the active vessel.
- Single Impulse { get; }¶
The impulse that the decoupler imparts when it is fired, in Newton seconds.
- Game Scenes:
All
Docking Port¶
- class DockingPort¶
A docking port. Obtained by calling
Part.DockingPort
- DockingPortState State { get; }¶
The current state of the docking port.
- Game Scenes:
All
- Part DockedPart { get; }¶
The part that this docking port is docked to. Returns
null
if this docking port is not docked to anything.- Game Scenes:
All
- Vessel Undock ()¶
Undocks the docking port and returns the new
Vessel
that is created. This method can be called for either docking port in a docked pair. Throws an exception if the docking port is not docked to anything.- Game Scenes:
All
Note
When called, the active vessel may change. It is therefore possible that, after calling this function, the object(s) returned by previous call(s) to
SpaceCenter.ActiveVessel
no longer refer to the active vessel.
- Single ReengageDistance { get; }¶
The distance a docking port must move away when it undocks before it becomes ready to dock with another port, in meters.
- Game Scenes:
All
- Boolean Shielded { get; set; }¶
The state of the docking ports shield, if it has one.
Returns
true
if the docking port has a shield, and the shield is closed. Otherwise returnsfalse
. When set totrue
, the shield is closed, and when set tofalse
the shield is opened. If the docking port does not have a shield, setting this attribute has no effect.- Game Scenes:
All
- Boolean CanRotate { get; }¶
Whether the docking port can be commanded to rotate while docked.
- Game Scenes:
All
- Boolean RotationLocked { get; set; }¶
Lock rotation. When locked, allows auto-strut to work across the joint.
- Game Scenes:
All
- Tuple<Double, Double, Double> Position (ReferenceFrame referenceFrame)¶
The position of the docking port, in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned position vector is in.
- Returns:
The position as a vector.
- Game Scenes:
All
- Tuple<Double, Double, Double> Direction (ReferenceFrame referenceFrame)¶
The direction that docking port points in, in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned direction is in.
- Returns:
The direction as a unit vector.
- Game Scenes:
All
- Tuple<Double, Double, Double, Double> Rotation (ReferenceFrame referenceFrame)¶
The rotation of the docking port, in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned rotation is in.
- Returns:
The rotation as a quaternion of the form \((x, y, z, w)\).
- Game Scenes:
All
- ReferenceFrame ReferenceFrame { get; }¶
The reference frame that is fixed relative to this docking port, and oriented with the port.
The origin is at the position of the docking port.
The axes rotate with the docking port.
The x-axis points out to the right side of the docking port.
The y-axis points in the direction the docking port is facing.
The z-axis points out of the bottom off the docking port.
- Game Scenes:
All
Note
This reference frame is not necessarily equivalent to the reference frame for the part, returned by
Part.ReferenceFrame
.Docking port reference frame origin and axes¶
Inline docking port reference frame origin and axes¶
- enum DockingPortState¶
The state of a docking port. See
DockingPort.State
.- Ready¶
The docking port is ready to dock to another docking port.
- Docked¶
The docking port is docked to another docking port, or docked to another part (from the VAB/SPH).
- Docking¶
The docking port is very close to another docking port, but has not docked. It is using magnetic force to acquire a solid dock.
- Undocking¶
The docking port has just been undocked from another docking port, and is disabled until it moves away by a sufficient distance (
DockingPort.ReengageDistance
).
- Shielded¶
The docking port has a shield, and the shield is closed.
- Moving¶
The docking ports shield is currently opening/closing.
Engine¶
- class Engine¶
An engine, including ones of various types. For example liquid fuelled gimballed engines, solid rocket boosters and jet engines. Obtained by calling
Part.Engine
.Note
For RCS thrusters
Part.RCS
.- Boolean Active { get; set; }¶
Whether the engine is active. Setting this attribute may have no effect, depending on
Engine.CanShutdown
andEngine.CanRestart
.- Game Scenes:
All
- Single Thrust { get; }¶
The current amount of thrust being produced by the engine, in Newtons.
- Game Scenes:
All
- Single AvailableThrust { get; }¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and with its throttle set to 100%. Returns zero if the engine does not have any fuel. Takes the engine’s current
Engine.ThrustLimit
and atmospheric conditions into account.- Game Scenes:
All
- Single AvailableThrustAt (Double pressure)¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and with its throttle set to 100%. Returns zero if the engine does not have any fuel. Takes the given pressure into account.
- Parameters:
pressure – Atmospheric pressure in atmospheres
- Game Scenes:
All
- Single MaxThrust { get; }¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and fueled, with its throttle and throttle limiter set to 100%.
- Game Scenes:
All
- Single MaxThrustAt (Double pressure)¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and fueled, with its throttle and throttle limiter set to 100%. Takes the given pressure into account.
- Parameters:
pressure – Atmospheric pressure in atmospheres
- Game Scenes:
All
- Single MaxVacuumThrust { get; }¶
The maximum amount of thrust that can be produced by the engine in a vacuum, in Newtons. This is the amount of thrust produced by the engine when activated,
Engine.ThrustLimit
is set to 100%, the main vessel’s throttle is set to 100% and the engine is in a vacuum.- Game Scenes:
All
- Single ThrustLimit { get; set; }¶
The thrust limiter of the engine. A value between 0 and 1. Setting this attribute may have no effect, for example the thrust limit for a solid rocket booster cannot be changed in flight.
- Game Scenes:
All
- IList<Thruster> Thrusters { get; }¶
The components of the engine that generate thrust.
- Game Scenes:
All
Note
For example, this corresponds to the rocket nozzel on a solid rocket booster, or the individual nozzels on a RAPIER engine. The overall thrust produced by the engine, as reported by
Engine.AvailableThrust
,Engine.MaxThrust
and others, is the sum of the thrust generated by each thruster.
- Single SpecificImpulse { get; }¶
The current specific impulse of the engine, in seconds. Returns zero if the engine is not active.
- Game Scenes:
All
- Single SpecificImpulseAt (Double pressure)¶
The specific impulse of the engine under the given pressure, in seconds. Returns zero if the engine is not active.
- Parameters:
pressure – Atmospheric pressure in atmospheres
- Game Scenes:
All
- Single VacuumSpecificImpulse { get; }¶
The vacuum specific impulse of the engine, in seconds.
- Game Scenes:
All
- Single KerbinSeaLevelSpecificImpulse { get; }¶
The specific impulse of the engine at sea level on Kerbin, in seconds.
- Game Scenes:
All
- IList<String> PropellantNames { get; }¶
The names of the propellants that the engine consumes.
- Game Scenes:
All
- IDictionary<String, Single> PropellantRatios { get; }¶
The ratio of resources that the engine consumes. A dictionary mapping resource names to the ratio at which they are consumed by the engine.
- Game Scenes:
All
Note
For example, if the ratios are 0.6 for LiquidFuel and 0.4 for Oxidizer, then for every 0.6 units of LiquidFuel that the engine burns, it will burn 0.4 units of Oxidizer.
- IList<Propellant> Propellants { get; }¶
The propellants that the engine consumes.
- Game Scenes:
All
- Single Throttle { get; set; }¶
The current throttle setting for the engine. A value between 0 and 1. This is not necessarily the same as the vessel’s main throttle setting, as some engines take time to adjust their throttle (such as jet engines), or independent throttle may be enabled.
When the engine’s independent throttle is enabled (see
Engine.IndependentThrottle
), can be used to set the throttle percentage.- Game Scenes:
All
- Boolean ThrottleLocked { get; }¶
Whether the
Control.Throttle
affects the engine. For example, this istrue
for liquid fueled rockets, andfalse
for solid rocket boosters.- Game Scenes:
All
- Boolean IndependentThrottle { get; set; }¶
Whether the independent throttle is enabled for the engine.
- Game Scenes:
All
- Boolean CanRestart { get; }¶
Whether the engine can be restarted once shutdown. If the engine cannot be shutdown, returns
false
. For example, this istrue
for liquid fueled rockets andfalse
for solid rocket boosters.- Game Scenes:
All
- Boolean CanShutdown { get; }¶
Whether the engine can be shutdown once activated. For example, this is
true
for liquid fueled rockets andfalse
for solid rocket boosters.- Game Scenes:
All
- IDictionary<String, Engine> Modes { get; }¶
The available modes for the engine. A dictionary mapping mode names to
Engine
objects.- Game Scenes:
All
- void ToggleMode ()¶
Toggle the current engine mode.
- Game Scenes:
All
- Boolean AutoModeSwitch { get; set; }¶
Whether the engine will automatically switch modes.
- Game Scenes:
All
- Single GimbalRange { get; }¶
The range over which the gimbal can move, in degrees. Returns 0 if the engine is not gimballed.
- Game Scenes:
All
- Boolean GimbalLocked { get; set; }¶
Whether the engines gimbal is locked in place. Setting this attribute has no effect if the engine is not gimballed.
- Game Scenes:
All
- Single GimbalLimit { get; set; }¶
The gimbal limiter of the engine. A value between 0 and 1. Returns 0 if the gimbal is locked.
- Game Scenes:
All
- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> AvailableTorque { get; }¶
The available torque, in Newton meters, that can be produced by this engine, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
Vessel.ReferenceFrame
. Returns zero if the engine is inactive, or not gimballed.- Game Scenes:
All
- class Propellant¶
A propellant for an engine. Obtains by calling
Engine.Propellants
.- Double TotalResourceAvailable { get; }¶
The total amount of the underlying resource currently reachable given resource flow rules.
- Game Scenes:
All
- Double TotalResourceCapacity { get; }¶
The total vehicle capacity for the underlying propellant resource, restricted by resource flow rules.
- Game Scenes:
All
- Boolean IgnoreForIsp { get; }¶
If this propellant should be ignored when calculating required mass flow given specific impulse.
- Game Scenes:
All
Experiment¶
- class Experiment¶
Obtained by calling
Part.Experiment
.- String Name { get; }¶
Internal name of the experiment, as used in part cfg files.
- Game Scenes:
All
- void Run ()¶
Run the experiment.
- Game Scenes:
All
- void Transmit ()¶
Transmit all experimental data contained by this part.
- Game Scenes:
All
- void Dump ()¶
Dump the experimental data contained by the experiment.
- Game Scenes:
All
- void Reset ()¶
Reset the experiment.
- Game Scenes:
All
- IList<ScienceData> Data { get; }¶
The data contained in this experiment.
- Game Scenes:
All
- Boolean Available { get; }¶
Determines if the experiment is available given the current conditions.
- Game Scenes:
All
- ScienceSubject ScienceSubject { get; }¶
Containing information on the corresponding specific science result for the current conditions. Returns
null
if the experiment is unavailable.- Game Scenes:
All
- class ScienceData¶
Obtained by calling
Experiment.Data
.
- class ScienceSubject¶
Obtained by calling
Experiment.ScienceSubject
.- Single Science { get; }¶
Amount of science already earned from this subject, not updated until after transmission/recovery.
- Game Scenes:
All
- Single DataScale { get; }¶
Multiply science value by this to determine data amount in mits.
- Game Scenes:
All
Fairing¶
- class Fairing¶
A fairing. Obtained by calling
Part.Fairing
. Supports both stock fairings, and those from the ProceduralFairings mod.- void Jettison ()¶
Jettison the fairing. Has no effect if it has already been jettisoned.
- Game Scenes:
All
Intake¶
- class Intake¶
An air intake. Obtained by calling
Part.Intake
.
Leg¶
Launch Clamp¶
- class LaunchClamp¶
A launch clamp. Obtained by calling
Part.LaunchClamp
.- void Release ()¶
Releases the docking clamp. Has no effect if the clamp has already been released.
- Game Scenes:
All
Light¶
Parachute¶
- class Parachute¶
A parachute. Obtained by calling
Part.Parachute
.- void Deploy ()¶
Deploys the parachute. This has no effect if the parachute has already been deployed.
- Game Scenes:
All
- void Arm ()¶
Deploys the parachute. This has no effect if the parachute has already been armed or deployed.
- Game Scenes:
All
- void Cut ()¶
Cuts the parachute.
- Game Scenes:
All
- ParachuteState State { get; }¶
The current state of the parachute.
- Game Scenes:
All
- enum ParachuteState¶
The state of a parachute. See
Parachute.State
.- Stowed¶
The parachute is safely tucked away inside its housing.
- Armed¶
The parachute is armed for deployment.
- SemiDeployed¶
The parachute has been deployed and is providing some drag, but is not fully deployed yet. (Stock parachutes only)
- Deployed¶
The parachute is fully deployed.
- Cut¶
The parachute has been cut.
Radiator¶
- class Radiator¶
A radiator. Obtained by calling
Part.Radiator
.- Boolean Deployed { get; set; }¶
For a deployable radiator,
true
if the radiator is extended. If the radiator is not deployable, this is alwaystrue
.- Game Scenes:
All
- RadiatorState State { get; }¶
The current state of the radiator.
- Game Scenes:
All
Note
A fixed radiator is always
RadiatorState.Extended
.
Resource Converter¶
- class ResourceConverter¶
A resource converter. Obtained by calling
Part.ResourceConverter
.- String Name (Int32 index)¶
The name of the specified converter.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- Boolean Active (Int32 index)¶
True if the specified converter is active.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- void Start (Int32 index)¶
Start the specified converter.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- void Stop (Int32 index)¶
Stop the specified converter.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- ResourceConverterState State (Int32 index)¶
The state of the specified converter.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- String StatusInfo (Int32 index)¶
Status information for the specified converter. This is the full status message shown in the in-game UI.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- IList<String> Inputs (Int32 index)¶
List of the names of resources consumed by the specified converter.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- IList<String> Outputs (Int32 index)¶
List of the names of resources produced by the specified converter.
- Parameters:
index – Index of the converter.
- Game Scenes:
All
- enum ResourceConverterState¶
The state of a resource converter. See
ResourceConverter.State
.- Running¶
Converter is running.
- Idle¶
Converter is idle.
- MissingResource¶
Converter is missing a required resource.
- StorageFull¶
No available storage for output resource.
- Capacity¶
At preset resource capacity.
- Unknown¶
Unknown state. Possible with modified resource converters. In this case, check
ResourceConverter.StatusInfo
for more information.
Resource Harvester¶
- class ResourceHarvester¶
A resource harvester (drill). Obtained by calling
Part.ResourceHarvester
.- ResourceHarvesterState State { get; }¶
The state of the harvester.
- Game Scenes:
All
- Single ExtractionRate { get; }¶
The rate at which the drill is extracting ore, in units per second.
- Game Scenes:
All
Reaction Wheel¶
- class ReactionWheel¶
A reaction wheel. Obtained by calling
Part.ReactionWheel
.- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> AvailableTorque { get; }¶
The available torque, in Newton meters, that can be produced by this reaction wheel, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
Vessel.ReferenceFrame
. Returns zero if the reaction wheel is inactive or broken.- Game Scenes:
All
- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> MaxTorque { get; }¶
The maximum torque, in Newton meters, that can be produced by this reaction wheel, when it is active, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
Vessel.ReferenceFrame
.- Game Scenes:
All
Resource Drain¶
- class ResourceDrain¶
A resource drain. Obtained by calling
Part.ResourceDrain
.- void SetResource (Resource resource, Boolean enabled)¶
Whether the given resource should be drained.
- Parameters:
- Game Scenes:
All
- Boolean CheckResource (Resource resource)¶
Whether the provided resource is enabled for draining.
- Parameters:
- Game Scenes:
All
- void Start ()¶
Activates resource draining for all enabled parts.
- Game Scenes:
All
- void Stop ()¶
Turns off resource draining.
- Game Scenes:
All
- enum DrainMode¶
Resource drain mode. See
ResourceDrain.DrainMode
.- Part¶
Drains from the parent part.
- Vessel¶
Drains from all available parts.
Robotic Controller¶
- class RoboticController¶
A robotic controller. Obtained by calling
Part.RoboticController
.- Boolean AddAxis (Module module, String fieldName)¶
Add an axis to the controller.
- Parameters:
- Returns:
Returns
true
if the axis is added successfully.- Game Scenes:
All
Robotic Hinge¶
- class RoboticHinge¶
A robotic hinge. Obtained by calling
Part.RoboticHinge
.- void MoveHome ()¶
Move hinge to it’s built position.
- Game Scenes:
All
Robotic Piston¶
- class RoboticPiston¶
A robotic piston part. Obtained by calling
Part.RoboticPiston
.- void MoveHome ()¶
Move piston to it’s built position.
- Game Scenes:
All
Robotic Rotation¶
- class RoboticRotation¶
A robotic rotation servo. Obtained by calling
Part.RoboticRotation
.- void MoveHome ()¶
Move rotation servo to it’s built position.
- Game Scenes:
All
Robotic Rotor¶
- class RoboticRotor¶
A robotic rotor. Obtained by calling
Part.RoboticRotor
.
RCS¶
- class RCS¶
An RCS block or thruster. Obtained by calling
Part.RCS
.- Boolean Active { get; }¶
Whether the RCS thrusters are active. An RCS thruster is inactive if the RCS action group is disabled (
Control.RCS
), the RCS thruster itself is not enabled (RCS.Enabled
) or it is covered by a fairing (Part.Shielded
).- Game Scenes:
All
- Boolean PitchEnabled { get; set; }¶
Whether the RCS thruster will fire when pitch control input is given.
- Game Scenes:
All
- Boolean YawEnabled { get; set; }¶
Whether the RCS thruster will fire when yaw control input is given.
- Game Scenes:
All
- Boolean RollEnabled { get; set; }¶
Whether the RCS thruster will fire when roll control input is given.
- Game Scenes:
All
- Boolean ForwardEnabled { get; set; }¶
Whether the RCS thruster will fire when pitch control input is given.
- Game Scenes:
All
- Boolean UpEnabled { get; set; }¶
Whether the RCS thruster will fire when yaw control input is given.
- Game Scenes:
All
- Boolean RightEnabled { get; set; }¶
Whether the RCS thruster will fire when roll control input is given.
- Game Scenes:
All
- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> AvailableTorque { get; }¶
The available torque, in Newton meters, that can be produced by this RCS, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
Vessel.ReferenceFrame
. Returns zero if RCS is disable.- Game Scenes:
All
- Tuple<Tuple<Double, Double, Double>, Tuple<Double, Double, Double>> AvailableForce { get; }¶
The available force, in Newtons, that can be produced by this RCS, in the positive and negative x, y and z axes of the vessel. These axes correspond to the coordinate axes of the
Vessel.ReferenceFrame
. Returns zero if RCS is disabled.- Game Scenes:
All
- Single AvailableThrust { get; }¶
The amount of thrust, in Newtons, that would be produced by the thruster when activated. Returns zero if the thruster does not have any fuel. Takes the thrusters current
RCS.ThrustLimit
and atmospheric conditions into account.- Game Scenes:
All
- Single MaxThrust { get; }¶
The maximum amount of thrust that can be produced by the RCS thrusters when active, in Newtons. Takes the thrusters current
RCS.ThrustLimit
and atmospheric conditions into account.- Game Scenes:
All
- Single MaxVacuumThrust { get; }¶
The maximum amount of thrust that can be produced by the RCS thrusters when active in a vacuum, in Newtons.
- Game Scenes:
All
- Single ThrustLimit { get; set; }¶
The thrust limiter of the thruster. A value between 0 and 1.
- Game Scenes:
All
- IList<Thruster> Thrusters { get; }¶
A list of thrusters, one of each nozzel in the RCS part.
- Game Scenes:
All
- Single SpecificImpulse { get; }¶
The current specific impulse of the RCS, in seconds. Returns zero if the RCS is not active.
- Game Scenes:
All
- Single VacuumSpecificImpulse { get; }¶
The vacuum specific impulse of the RCS, in seconds.
- Game Scenes:
All
- Single KerbinSeaLevelSpecificImpulse { get; }¶
The specific impulse of the RCS at sea level on Kerbin, in seconds.
- Game Scenes:
All
- IDictionary<String, Single> PropellantRatios { get; }¶
The ratios of resources that the RCS consumes. A dictionary mapping resource names to the ratios at which they are consumed by the RCS.
- Game Scenes:
All
Sensor¶
Solar Panel¶
- class SolarPanel¶
A solar panel. Obtained by calling
Part.SolarPanel
.- SolarPanelState State { get; }¶
The current state of the solar panel.
- Game Scenes:
All
Thruster¶
- class Thruster¶
The component of an
Engine
orRCS
part that generates thrust. Can obtained by callingEngine.Thrusters
orRCS.Thrusters
.Note
Engines can consist of multiple thrusters. For example, the S3 KS-25x4 “Mammoth” has four rocket nozzels, and so consists of four thrusters.
- Tuple<Double, Double, Double> ThrustPosition (ReferenceFrame referenceFrame)¶
The position at which the thruster generates thrust, in the given reference frame. For gimballed engines, this takes into account the current rotation of the gimbal.
- Parameters:
referenceFrame – The reference frame that the returned position vector is in.
- Returns:
The position as a vector.
- Game Scenes:
All
- Tuple<Double, Double, Double> ThrustDirection (ReferenceFrame referenceFrame)¶
The direction of the force generated by the thruster, in the given reference frame. This is opposite to the direction in which the thruster expels propellant. For gimballed engines, this takes into account the current rotation of the gimbal.
- Parameters:
referenceFrame – The reference frame that the returned direction is in.
- Returns:
The direction as a unit vector.
- Game Scenes:
All
- ReferenceFrame ThrustReferenceFrame { get; }¶
A reference frame that is fixed relative to the thruster and orientated with its thrust direction (
Thruster.ThrustDirection
). For gimballed engines, this takes into account the current rotation of the gimbal.The origin is at the position of thrust for this thruster (
Thruster.ThrustPosition
).The axes rotate with the thrust direction. This is the direction in which the thruster expels propellant, including any gimballing.
The y-axis points along the thrust direction.
The x-axis and z-axis are perpendicular to the thrust direction.
- Game Scenes:
All
- Tuple<Double, Double, Double> GimbalPosition (ReferenceFrame referenceFrame)¶
Position around which the gimbal pivots.
- Parameters:
referenceFrame – The reference frame that the returned position vector is in.
- Returns:
The position as a vector.
- Game Scenes:
All
- Tuple<Double, Double, Double> GimbalAngle { get; }¶
The current gimbal angle in the pitch, roll and yaw axes, in degrees.
- Game Scenes:
All
- Tuple<Double, Double, Double> InitialThrustPosition (ReferenceFrame referenceFrame)¶
The position at which the thruster generates thrust, when the engine is in its initial position (no gimballing), in the given reference frame.
- Parameters:
referenceFrame – The reference frame that the returned position vector is in.
- Returns:
The position as a vector.
- Game Scenes:
All
Note
This position can move when the gimbal rotates. This is because the thrust position and gimbal position are not necessarily the same.
- Tuple<Double, Double, Double> InitialThrustDirection (ReferenceFrame referenceFrame)¶
The direction of the force generated by the thruster, when the engine is in its initial position (no gimballing), in the given reference frame. This is opposite to the direction in which the thruster expels propellant.
- Parameters:
referenceFrame – The reference frame that the returned direction is in.
- Returns:
The direction as a unit vector.
- Game Scenes:
All
Wheel¶
- class Wheel¶
A wheel. Includes landing gear and rover wheels. Obtained by calling
Part.Wheel
. Can be used to control the motors, steering and deployment of wheels, among other things.- WheelState State { get; }¶
The current state of the wheel.
- Game Scenes:
All
- Single Brakes { get; set; }¶
The braking force, as a percentage of maximum, when the brakes are applied.
- Game Scenes:
All
- Boolean AutoFrictionControl { get; set; }¶
Whether automatic friction control is enabled.
- Game Scenes:
All
- Single ManualFrictionControl { get; set; }¶
Manual friction control value. Only has an effect if automatic friction control is disabled. A value between 0 and 5 inclusive.
- Game Scenes:
All
- Boolean MotorInverted { get; set; }¶
Whether the direction of the motor is inverted.
- Game Scenes:
All
- MotorState MotorState { get; }¶
Whether the direction of the motor is inverted.
- Game Scenes:
All
- Single MotorOutput { get; }¶
The output of the motor. This is the torque currently being generated, in Newton meters.
- Game Scenes:
All
- Boolean TractionControlEnabled { get; set; }¶
Whether automatic traction control is enabled. A wheel only has traction control if it is powered.
- Game Scenes:
All
- Single TractionControl { get; set; }¶
Setting for the traction control. Only takes effect if the wheel has automatic traction control enabled. A value between 0 and 5 inclusive.
- Game Scenes:
All
- Single DriveLimiter { get; set; }¶
Manual setting for the motor limiter. Only takes effect if the wheel has automatic traction control disabled. A value between 0 and 100 inclusive.
- Game Scenes:
All
- Single SuspensionSpringStrength { get; }¶
Suspension spring strength, as set in the editor.
- Game Scenes:
All
- Single SuspensionDamperStrength { get; }¶
Suspension damper strength, as set in the editor.
- Game Scenes:
All
Trees of Parts¶
Vessels in KSP are comprised of a number of parts, connected to one another in a
tree structure. An example vessel is shown in Figure 1, and the corresponding
tree of parts in Figure 2. The craft file for this example can also be
downloaded here
.
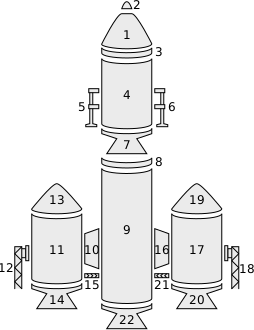
Figure 1 – Example parts making up a vessel.¶
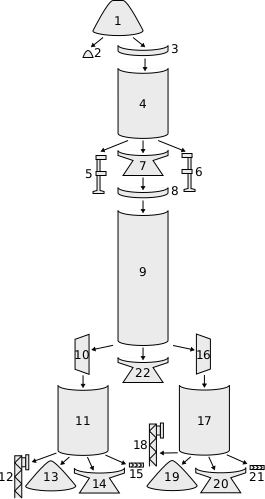
Figure 2 – Tree of parts for the vessel in Figure 1. Arrows point from the parent part to the child part.¶
Traversing the Tree¶
The tree of parts can be traversed using the attributes Parts.Root
,
Part.Parent
and Part.Children
.
The root of the tree is the same as the vessels root part (part number 1 in
the example above) and can be obtained by calling Parts.Root
.
A parts children can be obtained by calling Part.Children
.
If the part does not have any children, Part.Children
returns an empty list. A parts parent can be obtained by calling
Part.Parent
. If the part does not have a parent
(as is the case for the root part), Part.Parent
returns null
.
The following C# example uses these attributes to perform a depth-first traversal over all of the parts in a vessel:
using System;
using System.Collections.Generic;
using System.Net;
using KRPC.Client;
using KRPC.Client.Services.SpaceCenter;
class AttachmentModes
{
public static void Main ()
{
using (var connection = new Connection ()) {
var vessel = connection.SpaceCenter ().ActiveVessel;
var root = vessel.Parts.Root;
var stack = new Stack<Tuple<Part,int>> ();
stack.Push (new Tuple<Part,int> (root, 0));
while (stack.Count > 0) {
var item = stack.Pop ();
Part part = item.Item1;
int depth = item.Item2;
Console.WriteLine (new String (' ', depth) + part.Title);
foreach (var child in part.Children)
stack.Push (new Tuple<Part,int> (child, depth + 1));
}
}
}
}
When this code is execute using the craft file for the example vessel pictured above, the following is printed out:
Command Pod Mk1
TR-18A Stack Decoupler
FL-T400 Fuel Tank
LV-909 Liquid Fuel Engine
TR-18A Stack Decoupler
FL-T800 Fuel Tank
LV-909 Liquid Fuel Engine
TT-70 Radial Decoupler
FL-T400 Fuel Tank
TT18-A Launch Stability Enhancer
FTX-2 External Fuel Duct
LV-909 Liquid Fuel Engine
Aerodynamic Nose Cone
TT-70 Radial Decoupler
FL-T400 Fuel Tank
TT18-A Launch Stability Enhancer
FTX-2 External Fuel Duct
LV-909 Liquid Fuel Engine
Aerodynamic Nose Cone
LT-1 Landing Struts
LT-1 Landing Struts
Mk16 Parachute
Attachment Modes¶
Parts can be attached to other parts either radially (on the side of the parent part) or axially (on the end of the parent part, to form a stack).
For example, in the vessel pictured above, the parachute (part 2) is axially connected to its parent (the command pod – part 1), and the landing leg (part 5) is radially connected to its parent (the fuel tank – part 4).
The root part of a vessel (for example the command pod – part 1) does not have a parent part, so does not have an attachment mode. However, the part is consider to be axially attached to nothing.
The following C# example does a depth-first traversal as before, but also prints out the attachment mode used by the part:
using System;
using System.Collections.Generic;
using System.Net;
using KRPC.Client;
using KRPC.Client.Services.SpaceCenter;
class AttachmentModes
{
public static void Main ()
{
using (var connection = new Connection ()) {
var vessel = connection.SpaceCenter ().ActiveVessel;
var root = vessel.Parts.Root;
var stack = new Stack<Tuple<Part,int>> ();
stack.Push (new Tuple<Part,int> (root, 0));
while (stack.Count > 0) {
var item = stack.Pop ();
Part part = item.Item1;
int depth = item.Item2;
string attachMode = (part.AxiallyAttached ? "axial" : "radial");
Console.WriteLine (new String (' ', depth) + part.Title + " - " + attachMode);
foreach (var child in part.Children)
stack.Push (new Tuple<Part,int> (child, depth + 1));
}
}
}
}
When this code is execute using the craft file for the example vessel pictured above, the following is printed out:
Command Pod Mk1 - axial
TR-18A Stack Decoupler - axial
FL-T400 Fuel Tank - axial
LV-909 Liquid Fuel Engine - axial
TR-18A Stack Decoupler - axial
FL-T800 Fuel Tank - axial
LV-909 Liquid Fuel Engine - axial
TT-70 Radial Decoupler - radial
FL-T400 Fuel Tank - radial
TT18-A Launch Stability Enhancer - radial
FTX-2 External Fuel Duct - radial
LV-909 Liquid Fuel Engine - axial
Aerodynamic Nose Cone - axial
TT-70 Radial Decoupler - radial
FL-T400 Fuel Tank - radial
TT18-A Launch Stability Enhancer - radial
FTX-2 External Fuel Duct - radial
LV-909 Liquid Fuel Engine - axial
Aerodynamic Nose Cone - axial
LT-1 Landing Struts - radial
LT-1 Landing Struts - radial
Mk16 Parachute - axial
Fuel Lines¶
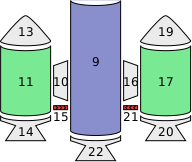
Figure 5 – Fuel lines from the example in Figure 1. Fuel flows from the parts highlighted in green, into the part highlighted in blue.¶
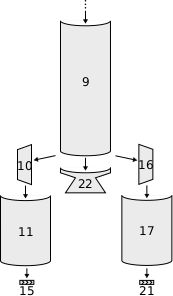
Figure 4 – A subset of the parts tree from Figure 2 above.¶
Fuel lines are considered parts, and are included in the parts tree (for example, as pictured in Figure 4). However, the parts tree does not contain information about which parts fuel lines connect to. The parent part of a fuel line is the part from which it will take fuel (as shown in Figure 4) however the part that it will send fuel to is not represented in the parts tree.
Figure 5 shows the fuel lines from the example vessel pictured earlier. Fuel line part 15 (in red) takes fuel from a fuel tank (part 11 – in green) and feeds it into another fuel tank (part 9 – in blue). The fuel line is therefore a child of part 11, but its connection to part 9 is not represented in the tree.
The attributes Part.FuelLinesFrom
and
Part.FuelLinesTo
can be used to discover these
connections. In the example in Figure 5, when
Part.FuelLinesTo
is called on fuel tank part
11, it will return a list of parts containing just fuel tank part 9 (the blue
part). When Part.FuelLinesFrom
is called on
fuel tank part 9, it will return a list containing fuel tank parts 11 and 17
(the parts colored green).
Staging¶
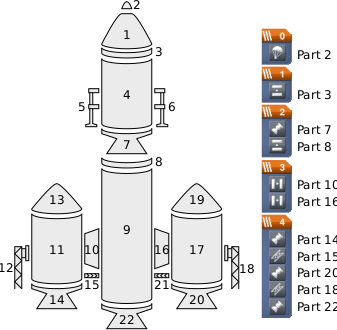
Figure 6 – Example vessel from Figure 1 with a staging sequence.¶
Each part has two staging numbers associated with it: the stage in which the
part is activated and the stage in which the part is decoupled. These values
can be obtained using Part.Stage
and
Part.DecoupleStage
respectively. For parts that
are not activated by staging, Part.Stage
returns
-1. For parts that are never decoupled,
Part.DecoupleStage
returns a value of -1.
Figure 6 shows an example staging sequence for a vessel. Figure 7 shows the stages in which each part of the vessel will be activated. Figure 8 shows the stages in which each part of the vessel will be decoupled.
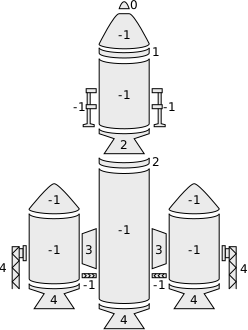
Figure 7 – The stage in which each part is activated.¶
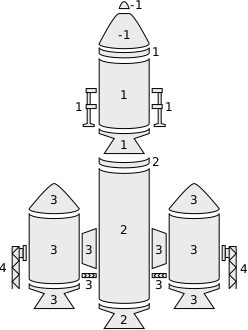
Figure 8 – The stage in which each part is decoupled.¶