Parts¶
The following classes allow interaction with a vessels individual parts.
Parts¶
- class SpaceCenter.Parts¶
Instances of this class are used to interact with the parts of a vessel. An instance can be obtained by calling
SpaceCenter.Vessel.parts
.- all: List¶
A list of all of the vessels parts.
- Attribute:
Read-only, cannot be set
- Return type:
List
- root: SpaceCenter.Part¶
The vessels root part.
- Attribute:
Read-only, cannot be set
- Return type:
Note
See the discussion on Trees of Parts.
- controlling: SpaceCenter.Part¶
The part from which the vessel is controlled.
- Attribute:
Can be read or written
- Return type:
- with_name(name)¶
A list of parts whose
SpaceCenter.Part.name
is name.- Parameters:
name (
string
) –- Return type:
List
- with_title(title)¶
A list of all parts whose
SpaceCenter.Part.title
is title.- Parameters:
title (
string
) –- Return type:
List
- with_tag(tag)¶
A list of all parts whose
SpaceCenter.Part.tag
is tag.- Parameters:
tag (
string
) –- Return type:
List
- with_module(module_name)¶
A list of all parts that contain a
SpaceCenter.Module
whoseSpaceCenter.Module.name
is module_name.- Parameters:
module_name (
string
) –- Return type:
List
- in_stage(stage)¶
A list of all parts that are activated in the given stage.
- Parameters:
stage (
number
) –- Return type:
List
Note
See the discussion on Staging.
- in_decouple_stage(stage)¶
A list of all parts that are decoupled in the given stage.
- Parameters:
stage (
number
) –- Return type:
List
Note
See the discussion on Staging.
- modules_with_name(module_name)¶
A list of modules (combined across all parts in the vessel) whose
SpaceCenter.Module.name
is module_name.- Parameters:
module_name (
string
) –- Return type:
List
- antennas: List¶
A list of all antennas in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
Note
If RemoteTech is installed, this will always return an empty list. To interact with RemoteTech antennas, use the RemoteTech service APIs.
- cargo_bays: List¶
A list of all cargo bays in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- control_surfaces: List¶
A list of all control surfaces in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- decouplers: List¶
A list of all decouplers in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- docking_ports: List¶
A list of all docking ports in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- engines: List¶
A list of all engines in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
Note
This includes any part that generates thrust. This covers many different types of engine, including liquid fuel rockets, solid rocket boosters, jet engines and RCS thrusters.
- experiments: List¶
A list of all science experiments in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- fairings: List¶
A list of all fairings in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- intakes: List¶
A list of all intakes in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- legs: List¶
A list of all landing legs attached to the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- launch_clamps: List¶
A list of all launch clamps attached to the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- lights: List¶
A list of all lights in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- parachutes: List¶
A list of all parachutes in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- radiators: List¶
A list of all radiators in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- resource_drains: List¶
A list of all resource drains in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- rcs: List¶
A list of all RCS blocks/thrusters in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- reaction_wheels: List¶
A list of all reaction wheels in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- resource_converters: List¶
A list of all resource converters in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- resource_harvesters: List¶
A list of all resource harvesters in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- robotic_hinges: List¶
A list of all robotic hinges in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- robotic_pistons: List¶
A list of all robotic pistons in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- robotic_rotations: List¶
A list of all robotic rotations in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- robotic_rotors: List¶
A list of all robotic rotors in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- sensors: List¶
A list of all sensors in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- solar_panels: List¶
A list of all solar panels in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
- wheels: List¶
A list of all wheels in the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
List
Part¶
- class SpaceCenter.Part¶
Represents an individual part. Vessels are made up of multiple parts. Instances of this class can be obtained by several methods in
SpaceCenter.Parts
.- name: string¶
Internal name of the part, as used in part cfg files. For example “Mark1-2Pod”.
- Attribute:
Read-only, cannot be set
- Return type:
string
- title: string¶
Title of the part, as shown when the part is right clicked in-game. For example “Mk1-2 Command Pod”.
- Attribute:
Read-only, cannot be set
- Return type:
string
- tag: string¶
The name tag for the part. Can be set to a custom string using the in-game user interface.
- Attribute:
Can be read or written
- Return type:
string
Note
This string is shared with kOS if it is installed.
- flag_url: string¶
The asset URL for the part’s flag.
- Attribute:
Can be read or written
- Return type:
string
- highlighted: boolean¶
Whether the part is highlighted.
- Attribute:
Can be read or written
- Return type:
boolean
- highlight_color: Tuple¶
The color used to highlight the part, as an RGB triple.
- Attribute:
Can be read or written
- Return type:
Tuple
- cost: number¶
The cost of the part, in units of funds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- vessel: SpaceCenter.Vessel¶
The vessel that contains this part.
- Attribute:
Read-only, cannot be set
- Return type:
- parent: SpaceCenter.Part¶
The parts parent. Returns
nil
if the part does not have a parent. This, in combination withSpaceCenter.Part.children
, can be used to traverse the vessels parts tree.- Attribute:
Read-only, cannot be set
- Return type:
Note
See the discussion on Trees of Parts.
- children: List¶
The parts children. Returns an empty list if the part has no children. This, in combination with
SpaceCenter.Part.parent
, can be used to traverse the vessels parts tree.- Attribute:
Read-only, cannot be set
- Return type:
List
Note
See the discussion on Trees of Parts.
- axially_attached: boolean¶
Whether the part is axially attached to its parent, i.e. on the top or bottom of its parent. If the part has no parent, returns
False
.- Attribute:
Read-only, cannot be set
- Return type:
boolean
Note
See the discussion on Attachment Modes.
- radially_attached: boolean¶
Whether the part is radially attached to its parent, i.e. on the side of its parent. If the part has no parent, returns
False
.- Attribute:
Read-only, cannot be set
- Return type:
boolean
Note
See the discussion on Attachment Modes.
- stage: number¶
The stage in which this part will be activated. Returns -1 if the part is not activated by staging.
- Attribute:
Read-only, cannot be set
- Return type:
number
Note
See the discussion on Staging.
- decouple_stage: number¶
The stage in which this part will be decoupled. Returns -1 if the part is never decoupled from the vessel.
- Attribute:
Read-only, cannot be set
- Return type:
number
Note
See the discussion on Staging.
- massless: boolean¶
Whether the part is massless.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- mass: number¶
The current mass of the part, including resources it contains, in kilograms. Returns zero if the part is massless.
- Attribute:
Read-only, cannot be set
- Return type:
number
- dry_mass: number¶
The mass of the part, not including any resources it contains, in kilograms. Returns zero if the part is massless.
- Attribute:
Read-only, cannot be set
- Return type:
number
- shielded: boolean¶
Whether the part is shielded from the exterior of the vessel, for example by a fairing.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- dynamic_pressure: number¶
The dynamic pressure acting on the part, in Pascals.
- Attribute:
Read-only, cannot be set
- Return type:
number
- impact_tolerance: number¶
The impact tolerance of the part, in meters per second.
- Attribute:
Read-only, cannot be set
- Return type:
number
- temperature: number¶
Temperature of the part, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- skin_temperature: number¶
Temperature of the skin of the part, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- max_temperature: number¶
Maximum temperature that the part can survive, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- max_skin_temperature: number¶
Maximum temperature that the skin of the part can survive, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_mass: number¶
A measure of how much energy it takes to increase the internal temperature of the part, in Joules per Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_skin_mass: number¶
A measure of how much energy it takes to increase the skin temperature of the part, in Joules per Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_resource_mass: number¶
A measure of how much energy it takes to increase the temperature of the resources contained in the part, in Joules per Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_conduction_flux: number¶
The rate at which heat energy is conducting into or out of the part via contact with other parts. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_convection_flux: number¶
The rate at which heat energy is convecting into or out of the part from the surrounding atmosphere. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_radiation_flux: number¶
The rate at which heat energy is radiating into or out of the part from the surrounding environment. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_internal_flux: number¶
The rate at which heat energy is begin generated by the part. For example, some engines generate heat by combusting fuel. Measured in energy per unit time, or power, in Watts. A positive value means the part is gaining heat energy, and negative means it is losing heat energy.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_skin_to_internal_flux: number¶
The rate at which heat energy is transferring between the part’s skin and its internals. Measured in energy per unit time, or power, in Watts. A positive value means the part’s internals are gaining heat energy, and negative means its skin is gaining heat energy.
- Attribute:
Read-only, cannot be set
- Return type:
number
- available_seats: number¶
How many open seats the part has.
- Attribute:
Read-only, cannot be set
- Return type:
number
- resources: SpaceCenter.Resources¶
A
SpaceCenter.Resources
object for the part.- Attribute:
Read-only, cannot be set
- Return type:
- crossfeed: boolean¶
Whether this part is crossfeed capable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- is_fuel_line: boolean¶
Whether this part is a fuel line.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- fuel_lines_from: List¶
The parts that are connected to this part via fuel lines, where the direction of the fuel line is into this part.
- Attribute:
Read-only, cannot be set
- Return type:
List
Note
See the discussion on Fuel Lines.
- fuel_lines_to: List¶
The parts that are connected to this part via fuel lines, where the direction of the fuel line is out of this part.
- Attribute:
Read-only, cannot be set
- Return type:
List
Note
See the discussion on Fuel Lines.
- modules: List¶
The modules for this part.
- Attribute:
Read-only, cannot be set
- Return type:
List
- antenna: SpaceCenter.Antenna¶
An
SpaceCenter.Antenna
if the part is an antenna, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
Note
If RemoteTech is installed, this will always return
nil
. To interact with RemoteTech antennas, use the RemoteTech service APIs.
- cargo_bay: SpaceCenter.CargoBay¶
A
SpaceCenter.CargoBay
if the part is a cargo bay, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- control_surface: SpaceCenter.ControlSurface¶
A
SpaceCenter.ControlSurface
if the part is an aerodynamic control surface, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- decoupler: SpaceCenter.Decoupler¶
A
SpaceCenter.Decoupler
if the part is a decoupler, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- docking_port: SpaceCenter.DockingPort¶
A
SpaceCenter.DockingPort
if the part is a docking port, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- engine: SpaceCenter.Engine¶
An
SpaceCenter.Engine
if the part is an engine, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- experiment: SpaceCenter.Experiment¶
An
SpaceCenter.Experiment
if the part contains a single science experiment, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
Note
Throws an exception if the part contains more than one experiment. In that case, use
SpaceCenter.Part.experiments
to get the list of experiments in the part.
- experiments: List¶
A list of
SpaceCenter.Experiment
objects that the part contains.- Attribute:
Read-only, cannot be set
- Return type:
List
- fairing: SpaceCenter.Fairing¶
A
SpaceCenter.Fairing
if the part is a fairing, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- intake: SpaceCenter.Intake¶
An
SpaceCenter.Intake
if the part is an intake, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
Note
This includes any part that generates thrust. This covers many different types of engine, including liquid fuel rockets, solid rocket boosters and jet engines. For RCS thrusters see
SpaceCenter.RCS
.
- leg: SpaceCenter.Leg¶
A
SpaceCenter.Leg
if the part is a landing leg, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- launch_clamp: SpaceCenter.LaunchClamp¶
A
SpaceCenter.LaunchClamp
if the part is a launch clamp, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- light: SpaceCenter.Light¶
A
SpaceCenter.Light
if the part is a light, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- parachute: SpaceCenter.Parachute¶
A
SpaceCenter.Parachute
if the part is a parachute, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- radiator: SpaceCenter.Radiator¶
A
SpaceCenter.Radiator
if the part is a radiator, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- resource_drain: SpaceCenter.ResourceDrain¶
A
SpaceCenter.ResourceDrain
if the part is a resource drain, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- rcs: SpaceCenter.RCS¶
A
SpaceCenter.RCS
if the part is an RCS block/thruster, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- reaction_wheel: SpaceCenter.ReactionWheel¶
A
SpaceCenter.ReactionWheel
if the part is a reaction wheel, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- resource_converter: SpaceCenter.ResourceConverter¶
A
SpaceCenter.ResourceConverter
if the part is a resource converter, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- resource_harvester: SpaceCenter.ResourceHarvester¶
A
SpaceCenter.ResourceHarvester
if the part is a resource harvester, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- robotic_controller: SpaceCenter.RoboticController¶
A
SpaceCenter.RoboticController
if the part is a robotic controller, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- robotic_hinge: SpaceCenter.RoboticHinge¶
A
SpaceCenter.RoboticHinge
if the part is a robotic hinge, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- robotic_piston: SpaceCenter.RoboticPiston¶
A
SpaceCenter.RoboticPiston
if the part is a robotic piston, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- robotic_rotation: SpaceCenter.RoboticRotation¶
A
SpaceCenter.RoboticRotation
if the part is a robotic rotation servo, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- robotic_rotor: SpaceCenter.RoboticRotor¶
A
SpaceCenter.RoboticRotor
if the part is a robotic rotor, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- sensor: SpaceCenter.Sensor¶
A
SpaceCenter.Sensor
if the part is a sensor, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- solar_panel: SpaceCenter.SolarPanel¶
A
SpaceCenter.SolarPanel
if the part is a solar panel, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- wheel: SpaceCenter.Wheel¶
A
SpaceCenter.Wheel
if the part is a wheel, otherwisenil
.- Attribute:
Read-only, cannot be set
- Return type:
- position(reference_frame)¶
The position of the part in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vector is in.- Returns:
The position as a vector.
- Return type:
Tuple
Note
This is a fixed position in the part, defined by the parts model. It s not necessarily the same as the parts center of mass. Use
SpaceCenter.Part.center_of_mass()
to get the parts center of mass.
- center_of_mass(reference_frame)¶
The position of the parts center of mass in the given reference frame. If the part is physicsless, this is equivalent to
SpaceCenter.Part.position()
.- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vector is in.- Returns:
The position as a vector.
- Return type:
Tuple
- bounding_box(reference_frame)¶
The axis-aligned bounding box of the part in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vectors are in.- Returns:
The positions of the minimum and maximum vertices of the box, as position vectors.
- Return type:
Tuple
Note
This is computed from the collision mesh of the part. If the part is not collidable, the box has zero volume and is centered on the
SpaceCenter.Part.position()
of the part.
- direction(reference_frame)¶
The direction the part points in, in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned direction is in.- Returns:
The direction as a unit vector.
- Return type:
Tuple
- velocity(reference_frame)¶
The linear velocity of the part in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned velocity vector is in.- Returns:
The velocity as a vector. The vector points in the direction of travel, and its magnitude is the speed of the body in meters per second.
- Return type:
Tuple
- rotation(reference_frame)¶
The rotation of the part, in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned rotation is in.- Returns:
The rotation as a quaternion of the form \((x, y, z, w)\).
- Return type:
Tuple
- moment_of_inertia: Tuple¶
The moment of inertia of the part in \(kg.m^2\) around its center of mass in the parts reference frame (
SpaceCenter.ReferenceFrame
).- Attribute:
Read-only, cannot be set
- Return type:
Tuple
- inertia_tensor: List¶
The inertia tensor of the part in the parts reference frame (
SpaceCenter.ReferenceFrame
). Returns the 3x3 matrix as a list of elements, in row-major order.- Attribute:
Read-only, cannot be set
- Return type:
List
- reference_frame: SpaceCenter.ReferenceFrame¶
The reference frame that is fixed relative to this part, and centered on a fixed position within the part, defined by the parts model.
The origin is at the position of the part, as returned by
SpaceCenter.Part.position()
.The axes rotate with the part.
The x, y and z axis directions depend on the design of the part.
- Attribute:
Read-only, cannot be set
- Return type:
Note
For docking port parts, this reference frame is not necessarily equivalent to the reference frame for the docking port, returned by
SpaceCenter.DockingPort.reference_frame
.Mk1 Command Pod reference frame origin and axes¶
- center_of_mass_reference_frame: SpaceCenter.ReferenceFrame¶
The reference frame that is fixed relative to this part, and centered on its center of mass.
The origin is at the center of mass of the part, as returned by
SpaceCenter.Part.center_of_mass()
.The axes rotate with the part.
The x, y and z axis directions depend on the design of the part.
- Attribute:
Read-only, cannot be set
- Return type:
Note
For docking port parts, this reference frame is not necessarily equivalent to the reference frame for the docking port, returned by
SpaceCenter.DockingPort.reference_frame
.
- add_force(force, position, reference_frame)¶
Exert a constant force on the part, acting at the given position.
- Parameters:
force (
Tuple
) – A vector pointing in the direction that the force acts, with its magnitude equal to the strength of the force in Newtons.position (
Tuple
) – The position at which the force acts, as a vector.reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the force and position are in.
- Returns:
An object that can be used to remove or modify the force.
- Return type:
- instantaneous_force(force, position, reference_frame)¶
Exert an instantaneous force on the part, acting at the given position.
- Parameters:
force (
Tuple
) – A vector pointing in the direction that the force acts, with its magnitude equal to the strength of the force in Newtons.position (
Tuple
) – The position at which the force acts, as a vector.reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the force and position are in.
Note
The force is applied instantaneously in a single physics update.
- glow: boolean¶
Whether the part is glowing.
- Attribute:
Write-only, cannot be read
- Return type:
boolean
- auto_strut_mode: SpaceCenter.AutoStrutMode¶
Auto-strut mode.
- Attribute:
Read-only, cannot be set
- Return type:
- class SpaceCenter.AutoStrutMode¶
The state of an auto-strut.
SpaceCenter.Part.auto_strut_mode
- off¶
Off
- root¶
Root
- heaviest¶
Heaviest
- grandparent¶
Grandparent
- force_root¶
ForceRoot
- force_heaviest¶
ForceHeaviest
- force_grandparent¶
ForceGrandparent
- class SpaceCenter.Force¶
Obtained by calling
SpaceCenter.Part.add_force()
.- part: SpaceCenter.Part¶
The part that this force is applied to.
- Attribute:
Read-only, cannot be set
- Return type:
- force_vector: Tuple¶
The force vector, in Newtons.
- Attribute:
Can be read or written
- Returns:
A vector pointing in the direction that the force acts, with its magnitude equal to the strength of the force in Newtons.
- Return type:
Tuple
- position: Tuple¶
The position at which the force acts, in reference frame
SpaceCenter.ReferenceFrame
.- Attribute:
Can be read or written
- Returns:
The position as a vector.
- Return type:
Tuple
- reference_frame: SpaceCenter.ReferenceFrame¶
The reference frame of the force vector and position.
- Attribute:
Can be read or written
- Return type:
- remove()¶
Remove the force.
Module¶
- class SpaceCenter.Module¶
This can be used to interact with a specific part module. This includes part modules in stock KSP, and those added by mods.
In KSP, each part has zero or more PartModules associated with it. Each one contains some of the functionality of the part. For example, an engine has a “ModuleEngines” part module that contains all the functionality of an engine.
- name: string¶
Name of the PartModule. For example, “ModuleEngines”.
- Attribute:
Read-only, cannot be set
- Return type:
string
- part: SpaceCenter.Part¶
The part that contains this module.
- Attribute:
Read-only, cannot be set
- Return type:
- fields: Map¶
The modules field names and their associated values, as a dictionary. These are the values visible in the right-click menu of the part.
- Attribute:
Read-only, cannot be set
- Return type:
Map
Note
Throws an exception if there is more than one field with the same name. In that case, use
SpaceCenter.Module.fields_by_id
to get the fields by identifier.
- fields_by_id: Map¶
The modules field identifiers and their associated values, as a dictionary. These are the values visible in the right-click menu of the part.
- Attribute:
Read-only, cannot be set
- Return type:
Map
- has_field(name)¶
Returns
True
if the module has a field with the given name.- Parameters:
name (
string
) – Name of the field.- Return type:
boolean
- has_field_with_id(id)¶
Returns
True
if the module has a field with the given identifier.- Parameters:
id (
string
) – Identifier of the field.- Return type:
boolean
- get_field(name)¶
Returns the value of a field with the given name.
- Parameters:
name (
string
) – Name of the field.- Return type:
string
- get_field_by_id(id)¶
Returns the value of a field with the given identifier.
- Parameters:
id (
string
) – Identifier of the field.- Return type:
string
- set_field_int(name, value)¶
Set the value of a field to the given integer number.
- Parameters:
name (
string
) – Name of the field.value (
number
) – Value to set.
- set_field_int_by_id(id, value)¶
Set the value of a field to the given integer number.
- Parameters:
id (
string
) – Identifier of the field.value (
number
) – Value to set.
- set_field_float(name, value)¶
Set the value of a field to the given floating point number.
- Parameters:
name (
string
) – Name of the field.value (
number
) – Value to set.
- set_field_float_by_id(id, value)¶
Set the value of a field to the given floating point number.
- Parameters:
id (
string
) – Identifier of the field.value (
number
) – Value to set.
- set_field_string(name, value)¶
Set the value of a field to the given string.
- Parameters:
name (
string
) – Name of the field.value (
string
) – Value to set.
- set_field_string_by_id(id, value)¶
Set the value of a field to the given string.
- Parameters:
id (
string
) – Identifier of the field.value (
string
) – Value to set.
- set_field_bool(name, value)¶
Set the value of a field to true or false.
- Parameters:
name (
string
) – Name of the field.value (
boolean
) – Value to set.
- set_field_bool_by_id(id, value)¶
Set the value of a field to true or false.
- Parameters:
id (
string
) – Identifier of the field.value (
boolean
) – Value to set.
- reset_field(name)¶
Set the value of a field to its original value.
- Parameters:
name (
string
) – Name of the field.
- reset_field_by_id(id)¶
Set the value of a field to its original value.
- Parameters:
id (
string
) – Identifier of the field.
- events: List¶
A list of the names of all of the modules events. Events are the clickable buttons visible in the right-click menu of the part.
- Attribute:
Read-only, cannot be set
- Return type:
List
- events_by_id: List¶
A list of the identifiers of all of the modules events. Events are the clickable buttons visible in the right-click menu of the part.
- Attribute:
Read-only, cannot be set
- Return type:
List
- has_event(name)¶
True
if the module has an event with the given name.- Parameters:
name (
string
) –- Return type:
boolean
- has_event_with_id(id)¶
True
if the module has an event with the given identifier.- Parameters:
id (
string
) –- Return type:
boolean
- trigger_event(name)¶
Trigger the named event. Equivalent to clicking the button in the right-click menu of the part.
- Parameters:
name (
string
) –
- trigger_event_by_id(id)¶
Trigger the event with the given identifier. Equivalent to clicking the button in the right-click menu of the part.
- Parameters:
id (
string
) –
- actions: List¶
A list of all the names of the modules actions. These are the parts actions that can be assigned to action groups in the in-game editor.
- Attribute:
Read-only, cannot be set
- Return type:
List
- actions_by_id: List¶
A list of all the identifiers of the modules actions. These are the parts actions that can be assigned to action groups in the in-game editor.
- Attribute:
Read-only, cannot be set
- Return type:
List
- has_action(name)¶
True
if the part has an action with the given name.- Parameters:
name (
string
) –- Return type:
boolean
- has_action_with_id(id)¶
True
if the part has an action with the given identifier.- Parameters:
id (
string
) –- Return type:
boolean
- set_action(name[, value = True])¶
Set the value of an action with the given name.
- Parameters:
name (
string
) –value (
boolean
) –
- set_action_by_id(id[, value = True])¶
Set the value of an action with the given identifier.
- Parameters:
id (
string
) –value (
boolean
) –
Specific Types of Part¶
The following classes provide functionality for specific types of part.
Antenna¶
Note
If RemoteTech is installed, use the RemoteTech service APIs to interact with antennas. This class is only for stock KSP antennas.
- class SpaceCenter.Antenna¶
An antenna. Obtained by calling
SpaceCenter.Part.antenna
.- part: SpaceCenter.Part¶
The part object for this antenna.
- Attribute:
Read-only, cannot be set
- Return type:
- state: SpaceCenter.AntennaState¶
The current state of the antenna.
- Attribute:
Read-only, cannot be set
- Return type:
- deployable: boolean¶
Whether the antenna is deployable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- deployed: boolean¶
Whether the antenna is deployed.
- Attribute:
Can be read or written
- Return type:
boolean
Note
Fixed antennas are always deployed. Returns an error if you try to deploy a fixed antenna.
- can_transmit: boolean¶
Whether data can be transmitted by this antenna.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- transmit()¶
Transmit data.
- cancel()¶
Cancel current transmission of data.
- allow_partial: boolean¶
Whether partial data transmission is permitted.
- Attribute:
Can be read or written
- Return type:
boolean
- power: number¶
The power of the antenna.
- Attribute:
Read-only, cannot be set
- Return type:
number
- combinable: boolean¶
Whether the antenna can be combined with other antennae on the vessel to boost the power.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- combinable_exponent: number¶
Exponent used to calculate the combined power of multiple antennae on a vessel.
- Attribute:
Read-only, cannot be set
- Return type:
number
- packet_interval: number¶
Interval between sending packets in seconds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- packet_size: number¶
Amount of data sent per packet in Mits.
- Attribute:
Read-only, cannot be set
- Return type:
number
- packet_resource_cost: number¶
Units of electric charge consumed per packet sent.
- Attribute:
Read-only, cannot be set
- Return type:
number
Cargo Bay¶
- class SpaceCenter.CargoBay¶
A cargo bay. Obtained by calling
SpaceCenter.Part.cargo_bay
.- part: SpaceCenter.Part¶
The part object for this cargo bay.
- Attribute:
Read-only, cannot be set
- Return type:
- state: SpaceCenter.CargoBayState¶
The state of the cargo bay.
- Attribute:
Read-only, cannot be set
- Return type:
- open: boolean¶
Whether the cargo bay is open.
- Attribute:
Can be read or written
- Return type:
boolean
Control Surface¶
- class SpaceCenter.ControlSurface¶
An aerodynamic control surface. Obtained by calling
SpaceCenter.Part.control_surface
.- part: SpaceCenter.Part¶
The part object for this control surface.
- Attribute:
Read-only, cannot be set
- Return type:
- pitch_enabled: boolean¶
Whether the control surface has pitch control enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- yaw_enabled: boolean¶
Whether the control surface has yaw control enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- roll_enabled: boolean¶
Whether the control surface has roll control enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- authority_limiter: number¶
The authority limiter for the control surface, which controls how far the control surface will move.
- Attribute:
Can be read or written
- Return type:
number
- inverted: boolean¶
Whether the control surface movement is inverted.
- Attribute:
Can be read or written
- Return type:
boolean
- deployed: boolean¶
Whether the control surface has been fully deployed.
- Attribute:
Can be read or written
- Return type:
boolean
- surface_area: number¶
Surface area of the control surface in \(m^2\).
- Attribute:
Read-only, cannot be set
- Return type:
number
- available_torque: Tuple¶
The available torque, in Newton meters, that can be produced by this control surface, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
SpaceCenter.Vessel.reference_frame
.- Attribute:
Read-only, cannot be set
- Return type:
Tuple
Decoupler¶
- class SpaceCenter.Decoupler¶
A decoupler. Obtained by calling
SpaceCenter.Part.decoupler
- part: SpaceCenter.Part¶
The part object for this decoupler.
- Attribute:
Read-only, cannot be set
- Return type:
- decouple()¶
Fires the decoupler. Returns the new vessel created when the decoupler fires. Throws an exception if the decoupler has already fired.
- Return type:
Note
When called, the active vessel may change. It is therefore possible that, after calling this function, the object(s) returned by previous call(s) to
SpaceCenter.active_vessel
no longer refer to the active vessel.
- decoupled: boolean¶
Whether the decoupler has fired.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- staged: boolean¶
Whether the decoupler is enabled in the staging sequence.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- impulse: number¶
The impulse that the decoupler imparts when it is fired, in Newton seconds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- is_omni_decoupler: boolean¶
Whether the decoupler is an omni-decoupler (e.g. stack separator)
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- attached_part: SpaceCenter.Part¶
The part attached to this decoupler’s explosive node.
- Attribute:
Read-only, cannot be set
- Return type:
Docking Port¶
- class SpaceCenter.DockingPort¶
A docking port. Obtained by calling
SpaceCenter.Part.docking_port
- part: SpaceCenter.Part¶
The part object for this docking port.
- Attribute:
Read-only, cannot be set
- Return type:
- state: SpaceCenter.DockingPortState¶
The current state of the docking port.
- Attribute:
Read-only, cannot be set
- Return type:
- docked_part: SpaceCenter.Part¶
The part that this docking port is docked to. Returns
nil
if this docking port is not docked to anything.- Attribute:
Read-only, cannot be set
- Return type:
- undock()¶
Undocks the docking port and returns the new
SpaceCenter.Vessel
that is created. This method can be called for either docking port in a docked pair. Throws an exception if the docking port is not docked to anything.- Return type:
Note
When called, the active vessel may change. It is therefore possible that, after calling this function, the object(s) returned by previous call(s) to
SpaceCenter.active_vessel
no longer refer to the active vessel.
- reengage_distance: number¶
The distance a docking port must move away when it undocks before it becomes ready to dock with another port, in meters.
- Attribute:
Read-only, cannot be set
- Return type:
number
- has_shield: boolean¶
Whether the docking port has a shield.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- shielded: boolean¶
The state of the docking ports shield, if it has one.
Returns
True
if the docking port has a shield, and the shield is closed. Otherwise returnsFalse
. When set toTrue
, the shield is closed, and when set toFalse
the shield is opened. If the docking port does not have a shield, setting this attribute has no effect.- Attribute:
Can be read or written
- Return type:
boolean
- can_rotate: boolean¶
Whether the docking port can be commanded to rotate while docked.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- maximum_rotation: number¶
Maximum rotation angle in degrees.
- Attribute:
Read-only, cannot be set
- Return type:
number
- minimum_rotation: number¶
Minimum rotation angle in degrees.
- Attribute:
Read-only, cannot be set
- Return type:
number
- rotation_target: number¶
Rotation target angle in degrees.
- Attribute:
Can be read or written
- Return type:
number
- rotation_locked: boolean¶
Lock rotation. When locked, allows auto-strut to work across the joint.
- Attribute:
Can be read or written
- Return type:
boolean
- position(reference_frame)¶
The position of the docking port, in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vector is in.- Returns:
The position as a vector.
- Return type:
Tuple
- direction(reference_frame)¶
The direction that docking port points in, in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned direction is in.- Returns:
The direction as a unit vector.
- Return type:
Tuple
- rotation(reference_frame)¶
The rotation of the docking port, in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned rotation is in.- Returns:
The rotation as a quaternion of the form \((x, y, z, w)\).
- Return type:
Tuple
- reference_frame: SpaceCenter.ReferenceFrame¶
The reference frame that is fixed relative to this docking port, and oriented with the port.
The origin is at the position of the docking port.
The axes rotate with the docking port.
The x-axis points out to the right side of the docking port.
The y-axis points in the direction the docking port is facing.
The z-axis points out of the bottom off the docking port.
- Attribute:
Read-only, cannot be set
- Return type:
Note
This reference frame is not necessarily equivalent to the reference frame for the part, returned by
SpaceCenter.Part.reference_frame
.Docking port reference frame origin and axes¶
Inline docking port reference frame origin and axes¶
- class SpaceCenter.DockingPortState¶
The state of a docking port. See
SpaceCenter.DockingPort.state
.- ready¶
The docking port is ready to dock to another docking port.
- docked¶
The docking port is docked to another docking port, or docked to another part (from the VAB/SPH).
- docking¶
The docking port is very close to another docking port, but has not docked. It is using magnetic force to acquire a solid dock.
- undocking¶
The docking port has just been undocked from another docking port, and is disabled until it moves away by a sufficient distance (
SpaceCenter.DockingPort.reengage_distance
).
- shielded¶
The docking port has a shield, and the shield is closed.
- moving¶
The docking ports shield is currently opening/closing.
Engine¶
- class SpaceCenter.Engine¶
An engine, including ones of various types. For example liquid fuelled gimballed engines, solid rocket boosters and jet engines. Obtained by calling
SpaceCenter.Part.engine
.Note
For RCS thrusters
SpaceCenter.Part.rcs
.- part: SpaceCenter.Part¶
The part object for this engine.
- Attribute:
Read-only, cannot be set
- Return type:
- active: boolean¶
Whether the engine is active. Setting this attribute may have no effect, depending on
SpaceCenter.Engine.can_shutdown
andSpaceCenter.Engine.can_restart
.- Attribute:
Can be read or written
- Return type:
boolean
- thrust: number¶
The current amount of thrust being produced by the engine, in Newtons.
- Attribute:
Read-only, cannot be set
- Return type:
number
- available_thrust: number¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and with its throttle set to 100%. Returns zero if the engine does not have any fuel. Takes the engine’s current
SpaceCenter.Engine.thrust_limit
and atmospheric conditions into account.- Attribute:
Read-only, cannot be set
- Return type:
number
- available_thrust_at(pressure)¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and with its throttle set to 100%. Returns zero if the engine does not have any fuel. Takes the given pressure into account.
- Parameters:
pressure (
number
) – Atmospheric pressure in atmospheres- Return type:
number
- max_thrust: number¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and fueled, with its throttle and throttle limiter set to 100%.
- Attribute:
Read-only, cannot be set
- Return type:
number
- max_thrust_at(pressure)¶
The amount of thrust, in Newtons, that would be produced by the engine when activated and fueled, with its throttle and throttle limiter set to 100%. Takes the given pressure into account.
- Parameters:
pressure (
number
) – Atmospheric pressure in atmospheres- Return type:
number
- max_vacuum_thrust: number¶
The maximum amount of thrust that can be produced by the engine in a vacuum, in Newtons. This is the amount of thrust produced by the engine when activated,
SpaceCenter.Engine.thrust_limit
is set to 100%, the main vessel’s throttle is set to 100% and the engine is in a vacuum.- Attribute:
Read-only, cannot be set
- Return type:
number
- thrust_limit: number¶
The thrust limiter of the engine. A value between 0 and 1. Setting this attribute may have no effect, for example the thrust limit for a solid rocket booster cannot be changed in flight.
- Attribute:
Can be read or written
- Return type:
number
- thrusters: List¶
The components of the engine that generate thrust.
- Attribute:
Read-only, cannot be set
- Return type:
List
Note
For example, this corresponds to the rocket nozzel on a solid rocket booster, or the individual nozzels on a RAPIER engine. The overall thrust produced by the engine, as reported by
SpaceCenter.Engine.available_thrust
,SpaceCenter.Engine.max_thrust
and others, is the sum of the thrust generated by each thruster.
- specific_impulse: number¶
The current specific impulse of the engine, in seconds. Returns zero if the engine is not active.
- Attribute:
Read-only, cannot be set
- Return type:
number
- specific_impulse_at(pressure)¶
The specific impulse of the engine under the given pressure, in seconds. Returns zero if the engine is not active.
- Parameters:
pressure (
number
) – Atmospheric pressure in atmospheres- Return type:
number
- vacuum_specific_impulse: number¶
The vacuum specific impulse of the engine, in seconds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- kerbin_sea_level_specific_impulse: number¶
The specific impulse of the engine at sea level on Kerbin, in seconds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- propellant_names: List¶
The names of the propellants that the engine consumes.
- Attribute:
Read-only, cannot be set
- Return type:
List
- propellant_ratios: Map¶
The ratio of resources that the engine consumes. A dictionary mapping resource names to the ratio at which they are consumed by the engine.
- Attribute:
Read-only, cannot be set
- Return type:
Map
Note
For example, if the ratios are 0.6 for LiquidFuel and 0.4 for Oxidizer, then for every 0.6 units of LiquidFuel that the engine burns, it will burn 0.4 units of Oxidizer.
- propellants: List¶
The propellants that the engine consumes.
- Attribute:
Read-only, cannot be set
- Return type:
List
- has_fuel: boolean¶
Whether the engine has any fuel available.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- throttle: number¶
The current throttle setting for the engine. A value between 0 and 1. This is not necessarily the same as the vessel’s main throttle setting, as some engines take time to adjust their throttle (such as jet engines), or independent throttle may be enabled.
When the engine’s independent throttle is enabled (see
SpaceCenter.Engine.independent_throttle
), can be used to set the throttle percentage.- Attribute:
Can be read or written
- Return type:
number
- throttle_locked: boolean¶
Whether the
SpaceCenter.Control.throttle
affects the engine. For example, this isTrue
for liquid fueled rockets, andFalse
for solid rocket boosters.- Attribute:
Read-only, cannot be set
- Return type:
boolean
- independent_throttle: boolean¶
Whether the independent throttle is enabled for the engine.
- Attribute:
Can be read or written
- Return type:
boolean
- can_restart: boolean¶
Whether the engine can be restarted once shutdown. If the engine cannot be shutdown, returns
False
. For example, this isTrue
for liquid fueled rockets andFalse
for solid rocket boosters.- Attribute:
Read-only, cannot be set
- Return type:
boolean
- can_shutdown: boolean¶
Whether the engine can be shutdown once activated. For example, this is
True
for liquid fueled rockets andFalse
for solid rocket boosters.- Attribute:
Read-only, cannot be set
- Return type:
boolean
- has_modes: boolean¶
Whether the engine has multiple modes of operation.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- mode: string¶
The name of the current engine mode.
- Attribute:
Can be read or written
- Return type:
string
- modes: Map¶
The available modes for the engine. A dictionary mapping mode names to
SpaceCenter.Engine
objects.- Attribute:
Read-only, cannot be set
- Return type:
Map
- toggle_mode()¶
Toggle the current engine mode.
- auto_mode_switch: boolean¶
Whether the engine will automatically switch modes.
- Attribute:
Can be read or written
- Return type:
boolean
- gimballed: boolean¶
Whether the engine is gimballed.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- gimbal_range: number¶
The range over which the gimbal can move, in degrees. Returns 0 if the engine is not gimballed.
- Attribute:
Read-only, cannot be set
- Return type:
number
- gimbal_locked: boolean¶
Whether the engines gimbal is locked in place. Setting this attribute has no effect if the engine is not gimballed.
- Attribute:
Can be read or written
- Return type:
boolean
- gimbal_limit: number¶
The gimbal limiter of the engine. A value between 0 and 1. Returns 0 if the gimbal is locked.
- Attribute:
Can be read or written
- Return type:
number
- available_torque: Tuple¶
The available torque, in Newton meters, that can be produced by this engine, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
SpaceCenter.Vessel.reference_frame
. Returns zero if the engine is inactive, or not gimballed.- Attribute:
Read-only, cannot be set
- Return type:
Tuple
- class SpaceCenter.Propellant¶
A propellant for an engine. Obtains by calling
SpaceCenter.Engine.propellants
.- name: string¶
The name of the propellant.
- Attribute:
Read-only, cannot be set
- Return type:
string
- current_amount: number¶
The current amount of propellant.
- Attribute:
Read-only, cannot be set
- Return type:
number
- current_requirement: number¶
The required amount of propellant.
- Attribute:
Read-only, cannot be set
- Return type:
number
- total_resource_available: number¶
The total amount of the underlying resource currently reachable given resource flow rules.
- Attribute:
Read-only, cannot be set
- Return type:
number
- total_resource_capacity: number¶
The total vehicle capacity for the underlying propellant resource, restricted by resource flow rules.
- Attribute:
Read-only, cannot be set
- Return type:
number
- ignore_for_isp: boolean¶
If this propellant should be ignored when calculating required mass flow given specific impulse.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- ignore_for_thrust_curve: boolean¶
If this propellant should be ignored for thrust curve calculations.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- draw_stack_gauge: boolean¶
If this propellant has a stack gauge or not.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- is_deprived: boolean¶
If this propellant is deprived.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- ratio: number¶
The propellant ratio.
- Attribute:
Read-only, cannot be set
- Return type:
number
Experiment¶
- class SpaceCenter.Experiment¶
Obtained by calling
SpaceCenter.Part.experiment
.- part: SpaceCenter.Part¶
The part object for this experiment.
- Attribute:
Read-only, cannot be set
- Return type:
- name: string¶
Internal name of the experiment, as used in part cfg files.
- Attribute:
Read-only, cannot be set
- Return type:
string
- title: string¶
Title of the experiment, as shown on the in-game UI.
- Attribute:
Read-only, cannot be set
- Return type:
string
- run()¶
Run the experiment.
- transmit()¶
Transmit all experimental data contained by this part.
- dump()¶
Dump the experimental data contained by the experiment.
- reset()¶
Reset the experiment.
- deployed: boolean¶
Whether the experiment has been deployed.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- rerunnable: boolean¶
Whether the experiment can be re-run.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- inoperable: boolean¶
Whether the experiment is inoperable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- has_data: boolean¶
Whether the experiment contains data.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- data: List¶
The data contained in this experiment.
- Attribute:
Read-only, cannot be set
- Return type:
List
- biome: string¶
The name of the biome the experiment is currently in.
- Attribute:
Read-only, cannot be set
- Return type:
string
- available: boolean¶
Determines if the experiment is available given the current conditions.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- science_subject: SpaceCenter.ScienceSubject¶
Containing information on the corresponding specific science result for the current conditions. Returns
nil
if the experiment is unavailable.- Attribute:
Read-only, cannot be set
- Return type:
- class SpaceCenter.ScienceData¶
Obtained by calling
SpaceCenter.Experiment.data
.- data_amount: number¶
Data amount.
- Attribute:
Read-only, cannot be set
- Return type:
number
- science_value: number¶
Science value.
- Attribute:
Read-only, cannot be set
- Return type:
number
- transmit_value: number¶
Transmit value.
- Attribute:
Read-only, cannot be set
- Return type:
number
- class SpaceCenter.ScienceSubject¶
Obtained by calling
SpaceCenter.Experiment.science_subject
.- title: string¶
Title of science subject, displayed in science archives
- Attribute:
Read-only, cannot be set
- Return type:
string
- is_complete: boolean¶
Whether the experiment has been completed.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- science: number¶
Amount of science already earned from this subject, not updated until after transmission/recovery.
- Attribute:
Read-only, cannot be set
- Return type:
number
- science_cap: number¶
Total science allowable for this subject.
- Attribute:
Read-only, cannot be set
- Return type:
number
- data_scale: number¶
Multiply science value by this to determine data amount in mits.
- Attribute:
Read-only, cannot be set
- Return type:
number
- subject_value: number¶
Multiplier for specific Celestial Body/Experiment Situation combination.
- Attribute:
Read-only, cannot be set
- Return type:
number
- scientific_value: number¶
Diminishing value multiplier for decreasing the science value returned from repeated experiments.
- Attribute:
Read-only, cannot be set
- Return type:
number
Fairing¶
- class SpaceCenter.Fairing¶
A fairing. Obtained by calling
SpaceCenter.Part.fairing
. Supports both stock fairings, and those from the ProceduralFairings mod.- part: SpaceCenter.Part¶
The part object for this fairing.
- Attribute:
Read-only, cannot be set
- Return type:
- jettison()¶
Jettison the fairing. Has no effect if it has already been jettisoned.
- jettisoned: boolean¶
Whether the fairing has been jettisoned.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
Intake¶
- class SpaceCenter.Intake¶
An air intake. Obtained by calling
SpaceCenter.Part.intake
.- part: SpaceCenter.Part¶
The part object for this intake.
- Attribute:
Read-only, cannot be set
- Return type:
- open: boolean¶
Whether the intake is open.
- Attribute:
Can be read or written
- Return type:
boolean
- speed: number¶
Speed of the flow into the intake, in \(m/s\).
- Attribute:
Read-only, cannot be set
- Return type:
number
- flow: number¶
The rate of flow into the intake, in units of resource per second.
- Attribute:
Read-only, cannot be set
- Return type:
number
- area: number¶
The area of the intake’s opening, in square meters.
- Attribute:
Read-only, cannot be set
- Return type:
number
Leg¶
- class SpaceCenter.Leg¶
A landing leg. Obtained by calling
SpaceCenter.Part.leg
.- part: SpaceCenter.Part¶
The part object for this landing leg.
- Attribute:
Read-only, cannot be set
- Return type:
- state: SpaceCenter.LegState¶
The current state of the landing leg.
- Attribute:
Read-only, cannot be set
- Return type:
- deployable: boolean¶
Whether the leg is deployable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- deployed: boolean¶
Whether the landing leg is deployed.
- Attribute:
Can be read or written
- Return type:
boolean
Note
Fixed landing legs are always deployed. Returns an error if you try to deploy fixed landing gear.
- is_grounded: boolean¶
Returns whether the leg is touching the ground.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
Launch Clamp¶
- class SpaceCenter.LaunchClamp¶
A launch clamp. Obtained by calling
SpaceCenter.Part.launch_clamp
.- part: SpaceCenter.Part¶
The part object for this launch clamp.
- Attribute:
Read-only, cannot be set
- Return type:
- release()¶
Releases the docking clamp. Has no effect if the clamp has already been released.
Light¶
- class SpaceCenter.Light¶
A light. Obtained by calling
SpaceCenter.Part.light
.- part: SpaceCenter.Part¶
The part object for this light.
- Attribute:
Read-only, cannot be set
- Return type:
- active: boolean¶
Whether the light is switched on.
- Attribute:
Can be read or written
- Return type:
boolean
- color: Tuple¶
The color of the light, as an RGB triple.
- Attribute:
Can be read or written
- Return type:
Tuple
- blink: boolean¶
Whether blinking is enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- blink_rate: number¶
The blink rate of the light.
- Attribute:
Can be read or written
- Return type:
number
- power_usage: number¶
The current power usage, in units of charge per second.
- Attribute:
Read-only, cannot be set
- Return type:
number
Parachute¶
- class SpaceCenter.Parachute¶
A parachute. Obtained by calling
SpaceCenter.Part.parachute
.- part: SpaceCenter.Part¶
The part object for this parachute.
- Attribute:
Read-only, cannot be set
- Return type:
- deploy()¶
Deploys the parachute. This has no effect if the parachute has already been deployed.
- deployed: boolean¶
Whether the parachute has been deployed.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- arm()¶
Deploys the parachute. This has no effect if the parachute has already been armed or deployed.
- armed: boolean¶
Whether the parachute has been armed or deployed.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- cut()¶
Cuts the parachute.
- state: SpaceCenter.ParachuteState¶
The current state of the parachute.
- Attribute:
Read-only, cannot be set
- Return type:
- deploy_altitude: number¶
The altitude at which the parachute will full deploy, in meters. Only applicable to stock parachutes.
- Attribute:
Can be read or written
- Return type:
number
- deploy_min_pressure: number¶
The minimum pressure at which the parachute will semi-deploy, in atmospheres. Only applicable to stock parachutes.
- Attribute:
Can be read or written
- Return type:
number
- class SpaceCenter.ParachuteState¶
The state of a parachute. See
SpaceCenter.Parachute.state
.- stowed¶
The parachute is safely tucked away inside its housing.
- armed¶
The parachute is armed for deployment.
- semi_deployed¶
The parachute has been deployed and is providing some drag, but is not fully deployed yet. (Stock parachutes only)
- deployed¶
The parachute is fully deployed.
- cut¶
The parachute has been cut.
Radiator¶
- class SpaceCenter.Radiator¶
A radiator. Obtained by calling
SpaceCenter.Part.radiator
.- part: SpaceCenter.Part¶
The part object for this radiator.
- Attribute:
Read-only, cannot be set
- Return type:
- deployable: boolean¶
Whether the radiator is deployable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- deployed: boolean¶
For a deployable radiator,
True
if the radiator is extended. If the radiator is not deployable, this is alwaysTrue
.- Attribute:
Can be read or written
- Return type:
boolean
- state: SpaceCenter.RadiatorState¶
The current state of the radiator.
- Attribute:
Read-only, cannot be set
- Return type:
Note
A fixed radiator is always
SpaceCenter.RadiatorState.extended
.
Resource Converter¶
- class SpaceCenter.ResourceConverter¶
A resource converter. Obtained by calling
SpaceCenter.Part.resource_converter
.- part: SpaceCenter.Part¶
The part object for this converter.
- Attribute:
Read-only, cannot be set
- Return type:
- count: number¶
The number of converters in the part.
- Attribute:
Read-only, cannot be set
- Return type:
number
- name(index)¶
The name of the specified converter.
- Parameters:
index (
number
) – Index of the converter.- Return type:
string
- active(index)¶
True if the specified converter is active.
- Parameters:
index (
number
) – Index of the converter.- Return type:
boolean
- start(index)¶
Start the specified converter.
- Parameters:
index (
number
) – Index of the converter.
- stop(index)¶
Stop the specified converter.
- Parameters:
index (
number
) – Index of the converter.
- state(index)¶
The state of the specified converter.
- Parameters:
index (
number
) – Index of the converter.- Return type:
- status_info(index)¶
Status information for the specified converter. This is the full status message shown in the in-game UI.
- Parameters:
index (
number
) – Index of the converter.- Return type:
string
- inputs(index)¶
List of the names of resources consumed by the specified converter.
- Parameters:
index (
number
) – Index of the converter.- Return type:
List
- outputs(index)¶
List of the names of resources produced by the specified converter.
- Parameters:
index (
number
) – Index of the converter.- Return type:
List
- optimum_core_temperature: number¶
The core temperature at which the converter will operate with peak efficiency, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- core_temperature: number¶
The core temperature of the converter, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_efficiency: number¶
The thermal efficiency of the converter, as a percentage of its maximum.
- Attribute:
Read-only, cannot be set
- Return type:
number
- class SpaceCenter.ResourceConverterState¶
The state of a resource converter. See
SpaceCenter.ResourceConverter.state()
.- running¶
Converter is running.
- idle¶
Converter is idle.
- missing_resource¶
Converter is missing a required resource.
- storage_full¶
No available storage for output resource.
- capacity¶
At preset resource capacity.
- unknown¶
Unknown state. Possible with modified resource converters. In this case, check
SpaceCenter.ResourceConverter.status_info()
for more information.
Resource Harvester¶
- class SpaceCenter.ResourceHarvester¶
A resource harvester (drill). Obtained by calling
SpaceCenter.Part.resource_harvester
.- part: SpaceCenter.Part¶
The part object for this harvester.
- Attribute:
Read-only, cannot be set
- Return type:
- state: SpaceCenter.ResourceHarvesterState¶
The state of the harvester.
- Attribute:
Read-only, cannot be set
- Return type:
- deployed: boolean¶
Whether the harvester is deployed.
- Attribute:
Can be read or written
- Return type:
boolean
- active: boolean¶
Whether the harvester is actively drilling.
- Attribute:
Can be read or written
- Return type:
boolean
- extraction_rate: number¶
The rate at which the drill is extracting ore, in units per second.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thermal_efficiency: number¶
The thermal efficiency of the drill, as a percentage of its maximum.
- Attribute:
Read-only, cannot be set
- Return type:
number
- core_temperature: number¶
The core temperature of the drill, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
- optimum_core_temperature: number¶
The core temperature at which the drill will operate with peak efficiency, in Kelvin.
- Attribute:
Read-only, cannot be set
- Return type:
number
Reaction Wheel¶
- class SpaceCenter.ReactionWheel¶
A reaction wheel. Obtained by calling
SpaceCenter.Part.reaction_wheel
.- part: SpaceCenter.Part¶
The part object for this reaction wheel.
- Attribute:
Read-only, cannot be set
- Return type:
- active: boolean¶
Whether the reaction wheel is active.
- Attribute:
Can be read or written
- Return type:
boolean
- broken: boolean¶
Whether the reaction wheel is broken.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- available_torque: Tuple¶
The available torque, in Newton meters, that can be produced by this reaction wheel, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
SpaceCenter.Vessel.reference_frame
. Returns zero if the reaction wheel is inactive or broken.- Attribute:
Read-only, cannot be set
- Return type:
Tuple
- max_torque: Tuple¶
The maximum torque, in Newton meters, that can be produced by this reaction wheel, when it is active, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
SpaceCenter.Vessel.reference_frame
.- Attribute:
Read-only, cannot be set
- Return type:
Tuple
Resource Drain¶
- class SpaceCenter.ResourceDrain¶
A resource drain. Obtained by calling
SpaceCenter.Part.resource_drain
.- part: SpaceCenter.Part¶
The part object for this resource drain.
- Attribute:
Read-only, cannot be set
- Return type:
- available_resources: List¶
List of available resources.
- Attribute:
Read-only, cannot be set
- Return type:
List
- set_resource(resource, enabled)¶
Whether the given resource should be drained.
- Parameters:
resource (
SpaceCenter.Resource
) –enabled (
boolean
) –
- check_resource(resource)¶
Whether the provided resource is enabled for draining.
- Parameters:
resource (
SpaceCenter.Resource
) –- Return type:
boolean
- drain_mode: SpaceCenter.DrainMode¶
The drain mode.
- Attribute:
Can be read or written
- Return type:
- min_rate: number¶
Minimum possible drain rate
- Attribute:
Read-only, cannot be set
- Return type:
number
- max_rate: number¶
Maximum possible drain rate.
- Attribute:
Read-only, cannot be set
- Return type:
number
- rate: number¶
Current drain rate.
- Attribute:
Can be read or written
- Return type:
number
- start()¶
Activates resource draining for all enabled parts.
- stop()¶
Turns off resource draining.
- class SpaceCenter.DrainMode¶
Resource drain mode. See
SpaceCenter.ResourceDrain.drain_mode
.- part¶
Drains from the parent part.
- vessel¶
Drains from all available parts.
Robotic Controller¶
- class SpaceCenter.RoboticController¶
A robotic controller. Obtained by calling
SpaceCenter.Part.robotic_controller
.- part: SpaceCenter.Part¶
The part object for this controller.
- Attribute:
Read-only, cannot be set
- Return type:
- has_part(part)¶
Whether the controller has a part.
- Parameters:
part (
SpaceCenter.Part
) –- Return type:
boolean
- axes()¶
The axes for the controller.
- Return type:
List
- add_axis(module, field_name)¶
Add an axis to the controller.
- Parameters:
module (
SpaceCenter.Module
) –field_name (
string
) –
- Returns:
Returns
True
if the axis is added successfully.- Return type:
boolean
- add_key_frame(module, field_name, time, value)¶
Add key frame value for controller axis.
- Parameters:
module (
SpaceCenter.Module
) –field_name (
string
) –time (
number
) –value (
number
) –
- Returns:
Returns
True
if the key frame is added successfully.- Return type:
boolean
- clear_axis(module, field_name)¶
Clear axis.
- Parameters:
module (
SpaceCenter.Module
) –field_name (
string
) –
- Returns:
Returns
True
if the axis is cleared successfully.- Return type:
boolean
Robotic Hinge¶
- class SpaceCenter.RoboticHinge¶
A robotic hinge. Obtained by calling
SpaceCenter.Part.robotic_hinge
.- part: SpaceCenter.Part¶
The part object for this robotic hinge.
- Attribute:
Read-only, cannot be set
- Return type:
- target_angle: number¶
Target angle.
- Attribute:
Can be read or written
- Return type:
number
- current_angle: number¶
Current angle.
- Attribute:
Read-only, cannot be set
- Return type:
number
- rate: number¶
Target movement rate in degrees per second.
- Attribute:
Can be read or written
- Return type:
number
- damping: number¶
Damping percentage.
- Attribute:
Can be read or written
- Return type:
number
- locked: boolean¶
Lock movement.
- Attribute:
Can be read or written
- Return type:
boolean
- motor_engaged: boolean¶
Whether the motor is engaged.
- Attribute:
Can be read or written
- Return type:
boolean
- move_home()¶
Move hinge to it’s built position.
Robotic Piston¶
- class SpaceCenter.RoboticPiston¶
A robotic piston part. Obtained by calling
SpaceCenter.Part.robotic_piston
.- part: SpaceCenter.Part¶
The part object for this robotic piston.
- Attribute:
Read-only, cannot be set
- Return type:
- target_extension: number¶
Target extension of the piston.
- Attribute:
Can be read or written
- Return type:
number
- current_extension: number¶
Current extension of the piston.
- Attribute:
Read-only, cannot be set
- Return type:
number
- rate: number¶
Target movement rate in degrees per second.
- Attribute:
Can be read or written
- Return type:
number
- damping: number¶
Damping percentage.
- Attribute:
Can be read or written
- Return type:
number
- locked: boolean¶
Lock movement.
- Attribute:
Can be read or written
- Return type:
boolean
- motor_engaged: boolean¶
Whether the motor is engaged.
- Attribute:
Can be read or written
- Return type:
boolean
- move_home()¶
Move piston to it’s built position.
Robotic Rotation¶
- class SpaceCenter.RoboticRotation¶
A robotic rotation servo. Obtained by calling
SpaceCenter.Part.robotic_rotation
.- part: SpaceCenter.Part¶
The part object for this robotic rotation servo.
- Attribute:
Read-only, cannot be set
- Return type:
- target_angle: number¶
Target angle.
- Attribute:
Can be read or written
- Return type:
number
- current_angle: number¶
Current angle.
- Attribute:
Read-only, cannot be set
- Return type:
number
- rate: number¶
Target movement rate in degrees per second.
- Attribute:
Can be read or written
- Return type:
number
- damping: number¶
Damping percentage.
- Attribute:
Can be read or written
- Return type:
number
- locked: boolean¶
Lock Movement
- Attribute:
Can be read or written
- Return type:
boolean
- motor_engaged: boolean¶
Whether the motor is engaged.
- Attribute:
Can be read or written
- Return type:
boolean
- move_home()¶
Move rotation servo to it’s built position.
Robotic Rotor¶
- class SpaceCenter.RoboticRotor¶
A robotic rotor. Obtained by calling
SpaceCenter.Part.robotic_rotor
.- part: SpaceCenter.Part¶
The part object for this robotic rotor.
- Attribute:
Read-only, cannot be set
- Return type:
- target_rpm: number¶
Target RPM.
- Attribute:
Can be read or written
- Return type:
number
- current_rpm: number¶
Current RPM.
- Attribute:
Read-only, cannot be set
- Return type:
number
- inverted: boolean¶
Whether the rotor direction is inverted.
- Attribute:
Can be read or written
- Return type:
boolean
- torque_limit: number¶
Torque limit percentage.
- Attribute:
Can be read or written
- Return type:
number
- locked: boolean¶
Lock movement.
- Attribute:
Can be read or written
- Return type:
boolean
- motor_engaged: boolean¶
Whether the motor is engaged.
- Attribute:
Can be read or written
- Return type:
boolean
RCS¶
- class SpaceCenter.RCS¶
An RCS block or thruster. Obtained by calling
SpaceCenter.Part.rcs
.- part: SpaceCenter.Part¶
The part object for this RCS.
- Attribute:
Read-only, cannot be set
- Return type:
- active: boolean¶
Whether the RCS thrusters are active. An RCS thruster is inactive if the RCS action group is disabled (
SpaceCenter.Control.rcs
), the RCS thruster itself is not enabled (SpaceCenter.RCS.enabled
) or it is covered by a fairing (SpaceCenter.Part.shielded
).- Attribute:
Read-only, cannot be set
- Return type:
boolean
- enabled: boolean¶
Whether the RCS thrusters are enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- pitch_enabled: boolean¶
Whether the RCS thruster will fire when pitch control input is given.
- Attribute:
Can be read or written
- Return type:
boolean
- yaw_enabled: boolean¶
Whether the RCS thruster will fire when yaw control input is given.
- Attribute:
Can be read or written
- Return type:
boolean
- roll_enabled: boolean¶
Whether the RCS thruster will fire when roll control input is given.
- Attribute:
Can be read or written
- Return type:
boolean
- forward_enabled: boolean¶
Whether the RCS thruster will fire when pitch control input is given.
- Attribute:
Can be read or written
- Return type:
boolean
- up_enabled: boolean¶
Whether the RCS thruster will fire when yaw control input is given.
- Attribute:
Can be read or written
- Return type:
boolean
- right_enabled: boolean¶
Whether the RCS thruster will fire when roll control input is given.
- Attribute:
Can be read or written
- Return type:
boolean
- available_torque: Tuple¶
The available torque, in Newton meters, that can be produced by this RCS, in the positive and negative pitch, roll and yaw axes of the vessel. These axes correspond to the coordinate axes of the
SpaceCenter.Vessel.reference_frame
. Returns zero if RCS is disable.- Attribute:
Read-only, cannot be set
- Return type:
Tuple
- available_force: Tuple¶
The available force, in Newtons, that can be produced by this RCS, in the positive and negative x, y and z axes of the vessel. These axes correspond to the coordinate axes of the
SpaceCenter.Vessel.reference_frame
. Returns zero if RCS is disabled.- Attribute:
Read-only, cannot be set
- Return type:
Tuple
- available_thrust: number¶
The amount of thrust, in Newtons, that would be produced by the thruster when activated. Returns zero if the thruster does not have any fuel. Takes the thrusters current
SpaceCenter.RCS.thrust_limit
and atmospheric conditions into account.- Attribute:
Read-only, cannot be set
- Return type:
number
- max_thrust: number¶
The maximum amount of thrust that can be produced by the RCS thrusters when active, in Newtons. Takes the thrusters current
SpaceCenter.RCS.thrust_limit
and atmospheric conditions into account.- Attribute:
Read-only, cannot be set
- Return type:
number
- max_vacuum_thrust: number¶
The maximum amount of thrust that can be produced by the RCS thrusters when active in a vacuum, in Newtons.
- Attribute:
Read-only, cannot be set
- Return type:
number
- thrust_limit: number¶
The thrust limiter of the thruster. A value between 0 and 1.
- Attribute:
Can be read or written
- Return type:
number
- thrusters: List¶
A list of thrusters, one of each nozzel in the RCS part.
- Attribute:
Read-only, cannot be set
- Return type:
List
- specific_impulse: number¶
The current specific impulse of the RCS, in seconds. Returns zero if the RCS is not active.
- Attribute:
Read-only, cannot be set
- Return type:
number
- vacuum_specific_impulse: number¶
The vacuum specific impulse of the RCS, in seconds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- kerbin_sea_level_specific_impulse: number¶
The specific impulse of the RCS at sea level on Kerbin, in seconds.
- Attribute:
Read-only, cannot be set
- Return type:
number
- propellants: List¶
The names of resources that the RCS consumes.
- Attribute:
Read-only, cannot be set
- Return type:
List
- propellant_ratios: Map¶
The ratios of resources that the RCS consumes. A dictionary mapping resource names to the ratios at which they are consumed by the RCS.
- Attribute:
Read-only, cannot be set
- Return type:
Map
- has_fuel: boolean¶
Whether the RCS has fuel available.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
Sensor¶
- class SpaceCenter.Sensor¶
A sensor, such as a thermometer. Obtained by calling
SpaceCenter.Part.sensor
.- part: SpaceCenter.Part¶
The part object for this sensor.
- Attribute:
Read-only, cannot be set
- Return type:
- active: boolean¶
Whether the sensor is active.
- Attribute:
Can be read or written
- Return type:
boolean
- value: string¶
The current value of the sensor.
- Attribute:
Read-only, cannot be set
- Return type:
string
Solar Panel¶
- class SpaceCenter.SolarPanel¶
A solar panel. Obtained by calling
SpaceCenter.Part.solar_panel
.- part: SpaceCenter.Part¶
The part object for this solar panel.
- Attribute:
Read-only, cannot be set
- Return type:
- deployable: boolean¶
Whether the solar panel is deployable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- deployed: boolean¶
Whether the solar panel is extended.
- Attribute:
Can be read or written
- Return type:
boolean
- state: SpaceCenter.SolarPanelState¶
The current state of the solar panel.
- Attribute:
Read-only, cannot be set
- Return type:
- energy_flow: number¶
The current amount of energy being generated by the solar panel, in units of charge per second.
- Attribute:
Read-only, cannot be set
- Return type:
number
- sun_exposure: number¶
The current amount of sunlight that is incident on the solar panel, as a percentage. A value between 0 and 1.
- Attribute:
Read-only, cannot be set
- Return type:
number
Thruster¶
- class SpaceCenter.Thruster¶
The component of an
SpaceCenter.Engine
orSpaceCenter.RCS
part that generates thrust. Can obtained by callingSpaceCenter.Engine.thrusters
orSpaceCenter.RCS.thrusters
.Note
Engines can consist of multiple thrusters. For example, the S3 KS-25x4 “Mammoth” has four rocket nozzels, and so consists of four thrusters.
- part: SpaceCenter.Part¶
The
SpaceCenter.Part
that contains this thruster.- Attribute:
Read-only, cannot be set
- Return type:
- thrust_position(reference_frame)¶
The position at which the thruster generates thrust, in the given reference frame. For gimballed engines, this takes into account the current rotation of the gimbal.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vector is in.- Returns:
The position as a vector.
- Return type:
Tuple
- thrust_direction(reference_frame)¶
The direction of the force generated by the thruster, in the given reference frame. This is opposite to the direction in which the thruster expels propellant. For gimballed engines, this takes into account the current rotation of the gimbal.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned direction is in.- Returns:
The direction as a unit vector.
- Return type:
Tuple
- thrust_reference_frame: SpaceCenter.ReferenceFrame¶
A reference frame that is fixed relative to the thruster and orientated with its thrust direction (
SpaceCenter.Thruster.thrust_direction()
). For gimballed engines, this takes into account the current rotation of the gimbal.The origin is at the position of thrust for this thruster (
SpaceCenter.Thruster.thrust_position()
).The axes rotate with the thrust direction. This is the direction in which the thruster expels propellant, including any gimballing.
The y-axis points along the thrust direction.
The x-axis and z-axis are perpendicular to the thrust direction.
- Attribute:
Read-only, cannot be set
- Return type:
- gimballed: boolean¶
Whether the thruster is gimballed.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- gimbal_position(reference_frame)¶
Position around which the gimbal pivots.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vector is in.- Returns:
The position as a vector.
- Return type:
Tuple
- gimbal_angle: Tuple¶
The current gimbal angle in the pitch, roll and yaw axes, in degrees.
- Attribute:
Read-only, cannot be set
- Return type:
Tuple
- initial_thrust_position(reference_frame)¶
The position at which the thruster generates thrust, when the engine is in its initial position (no gimballing), in the given reference frame.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned position vector is in.- Returns:
The position as a vector.
- Return type:
Tuple
Note
This position can move when the gimbal rotates. This is because the thrust position and gimbal position are not necessarily the same.
- initial_thrust_direction(reference_frame)¶
The direction of the force generated by the thruster, when the engine is in its initial position (no gimballing), in the given reference frame. This is opposite to the direction in which the thruster expels propellant.
- Parameters:
reference_frame (
SpaceCenter.ReferenceFrame
) – The reference frame that the returned direction is in.- Returns:
The direction as a unit vector.
- Return type:
Tuple
Wheel¶
- class SpaceCenter.Wheel¶
A wheel. Includes landing gear and rover wheels. Obtained by calling
SpaceCenter.Part.wheel
. Can be used to control the motors, steering and deployment of wheels, among other things.- part: SpaceCenter.Part¶
The part object for this wheel.
- Attribute:
Read-only, cannot be set
- Return type:
- state: SpaceCenter.WheelState¶
The current state of the wheel.
- Attribute:
Read-only, cannot be set
- Return type:
- radius: number¶
Radius of the wheel, in meters.
- Attribute:
Read-only, cannot be set
- Return type:
number
- grounded: boolean¶
Whether the wheel is touching the ground.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- has_brakes: boolean¶
Whether the wheel has brakes.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- brakes: number¶
The braking force, as a percentage of maximum, when the brakes are applied.
- Attribute:
Can be read or written
- Return type:
number
- auto_friction_control: boolean¶
Whether automatic friction control is enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- manual_friction_control: number¶
Manual friction control value. Only has an effect if automatic friction control is disabled. A value between 0 and 5 inclusive.
- Attribute:
Can be read or written
- Return type:
number
- deployable: boolean¶
Whether the wheel is deployable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- deployed: boolean¶
Whether the wheel is deployed.
- Attribute:
Can be read or written
- Return type:
boolean
- powered: boolean¶
Whether the wheel is powered by a motor.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- motor_enabled: boolean¶
Whether the motor is enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- motor_inverted: boolean¶
Whether the direction of the motor is inverted.
- Attribute:
Can be read or written
- Return type:
boolean
- motor_state: SpaceCenter.MotorState¶
Whether the direction of the motor is inverted.
- Attribute:
Read-only, cannot be set
- Return type:
- motor_output: number¶
The output of the motor. This is the torque currently being generated, in Newton meters.
- Attribute:
Read-only, cannot be set
- Return type:
number
- traction_control_enabled: boolean¶
Whether automatic traction control is enabled. A wheel only has traction control if it is powered.
- Attribute:
Can be read or written
- Return type:
boolean
- traction_control: number¶
Setting for the traction control. Only takes effect if the wheel has automatic traction control enabled. A value between 0 and 5 inclusive.
- Attribute:
Can be read or written
- Return type:
number
- drive_limiter: number¶
Manual setting for the motor limiter. Only takes effect if the wheel has automatic traction control disabled. A value between 0 and 100 inclusive.
- Attribute:
Can be read or written
- Return type:
number
- steerable: boolean¶
Whether the wheel has steering.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- steering_enabled: boolean¶
Whether the wheel steering is enabled.
- Attribute:
Can be read or written
- Return type:
boolean
- steering_inverted: boolean¶
Whether the wheel steering is inverted.
- Attribute:
Can be read or written
- Return type:
boolean
- steering_angle_limit: number¶
The steering angle limit.
- Attribute:
Can be read or written
- Return type:
number
- steering_response_time: number¶
Steering response time.
- Attribute:
Can be read or written
- Return type:
number
- has_suspension: boolean¶
Whether the wheel has suspension.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- suspension_spring_strength: number¶
Suspension spring strength, as set in the editor.
- Attribute:
Read-only, cannot be set
- Return type:
number
- suspension_damper_strength: number¶
Suspension damper strength, as set in the editor.
- Attribute:
Read-only, cannot be set
- Return type:
number
- broken: boolean¶
Whether the wheel is broken.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- repairable: boolean¶
Whether the wheel is repairable.
- Attribute:
Read-only, cannot be set
- Return type:
boolean
- stress: number¶
Current stress on the wheel.
- Attribute:
Read-only, cannot be set
- Return type:
number
- stress_tolerance: number¶
Stress tolerance of the wheel.
- Attribute:
Read-only, cannot be set
- Return type:
number
- stress_percentage: number¶
Current stress on the wheel as a percentage of its stress tolerance.
- Attribute:
Read-only, cannot be set
- Return type:
number
- deflection: number¶
Current deflection of the wheel.
- Attribute:
Read-only, cannot be set
- Return type:
number
- slip: number¶
Current slip of the wheel.
- Attribute:
Read-only, cannot be set
- Return type:
number
- class SpaceCenter.WheelState¶
The state of a wheel. See
SpaceCenter.Wheel.state
.- deployed¶
Wheel is fully deployed.
- retracted¶
Wheel is fully retracted.
- deploying¶
Wheel is being deployed.
- retracting¶
Wheel is being retracted.
- broken¶
Wheel is broken.
- class SpaceCenter.MotorState¶
The state of the motor on a powered wheel. See
SpaceCenter.Wheel.motor_state
.- idle¶
The motor is idle.
- running¶
The motor is running.
- disabled¶
The motor is disabled.
- inoperable¶
The motor is inoperable.
- not_enough_resources¶
The motor does not have enough resources to run.
Trees of Parts¶
Vessels in KSP are comprised of a number of parts, connected to one another in a
tree structure. An example vessel is shown in Figure 1, and the corresponding
tree of parts in Figure 2. The craft file for this example can also be
downloaded here
.
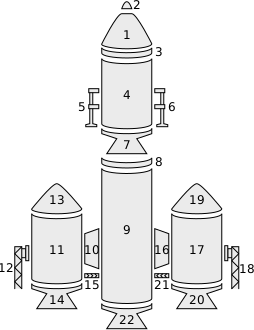
Figure 1 – Example parts making up a vessel.¶
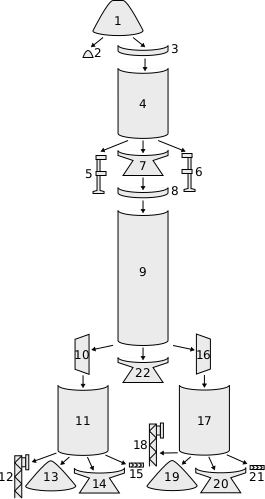
Figure 2 – Tree of parts for the vessel in Figure 1. Arrows point from the parent part to the child part.¶
Traversing the Tree¶
The tree of parts can be traversed using the attributes SpaceCenter.Parts.root
,
SpaceCenter.Part.parent
and SpaceCenter.Part.children
.
The root of the tree is the same as the vessels root part (part number 1 in
the example above) and can be obtained by calling SpaceCenter.Parts.root
.
A parts children can be obtained by calling SpaceCenter.Part.children
.
If the part does not have any children, SpaceCenter.Part.children
returns an empty list. A parts parent can be obtained by calling
SpaceCenter.Part.parent
. If the part does not have a parent
(as is the case for the root part), SpaceCenter.Part.parent
returns nil
.
The following Lua example uses these attributes to perform a depth-first traversal over all of the parts in a vessel:
local krpc = require 'krpc'
local conn = krpc.connect()
local vessel = conn.space_center.active_vessel
local root = vessel.parts.root
local stack = {{root,0}}
while #stack > 0 do
local part,depth = unpack(table.remove(stack))
print(string.rep(' ', depth) .. part.title)
for _,child in ipairs(part.children) do
table.insert(stack, {child, depth+1})
end
end
When this code is execute using the craft file for the example vessel pictured above, the following is printed out:
Command Pod Mk1
TR-18A Stack Decoupler
FL-T400 Fuel Tank
LV-909 Liquid Fuel Engine
TR-18A Stack Decoupler
FL-T800 Fuel Tank
LV-909 Liquid Fuel Engine
TT-70 Radial Decoupler
FL-T400 Fuel Tank
TT18-A Launch Stability Enhancer
FTX-2 External Fuel Duct
LV-909 Liquid Fuel Engine
Aerodynamic Nose Cone
TT-70 Radial Decoupler
FL-T400 Fuel Tank
TT18-A Launch Stability Enhancer
FTX-2 External Fuel Duct
LV-909 Liquid Fuel Engine
Aerodynamic Nose Cone
LT-1 Landing Struts
LT-1 Landing Struts
Mk16 Parachute
Attachment Modes¶
Parts can be attached to other parts either radially (on the side of the parent part) or axially (on the end of the parent part, to form a stack).
For example, in the vessel pictured above, the parachute (part 2) is axially connected to its parent (the command pod – part 1), and the landing leg (part 5) is radially connected to its parent (the fuel tank – part 4).
The root part of a vessel (for example the command pod – part 1) does not have a parent part, so does not have an attachment mode. However, the part is consider to be axially attached to nothing.
The following Lua example does a depth-first traversal as before, but also prints out the attachment mode used by the part:
local krpc = require 'krpc'
local conn = krpc.connect()
local vessel = conn.space_center.active_vessel
local root = vessel.parts.root
local stack = {{root, 0}}
while #stack > 0 do
local part,depth = unpack(table.remove(stack))
local attach_mode
if part.axially_attached then
attach_mode = 'axial'
else -- radially_attached
attach_mode = 'radial'
end
print(string.rep(' ', depth) .. part.title .. ' - ' .. attach_mode)
for _,child in ipairs(part.children) do
table.insert(stack, {child, depth+1})
end
end
When this code is execute using the craft file for the example vessel pictured above, the following is printed out:
Command Pod Mk1 - axial
TR-18A Stack Decoupler - axial
FL-T400 Fuel Tank - axial
LV-909 Liquid Fuel Engine - axial
TR-18A Stack Decoupler - axial
FL-T800 Fuel Tank - axial
LV-909 Liquid Fuel Engine - axial
TT-70 Radial Decoupler - radial
FL-T400 Fuel Tank - radial
TT18-A Launch Stability Enhancer - radial
FTX-2 External Fuel Duct - radial
LV-909 Liquid Fuel Engine - axial
Aerodynamic Nose Cone - axial
TT-70 Radial Decoupler - radial
FL-T400 Fuel Tank - radial
TT18-A Launch Stability Enhancer - radial
FTX-2 External Fuel Duct - radial
LV-909 Liquid Fuel Engine - axial
Aerodynamic Nose Cone - axial
LT-1 Landing Struts - radial
LT-1 Landing Struts - radial
Mk16 Parachute - axial
Fuel Lines¶
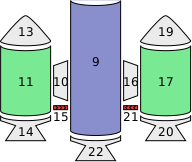
Figure 5 – Fuel lines from the example in Figure 1. Fuel flows from the parts highlighted in green, into the part highlighted in blue.¶
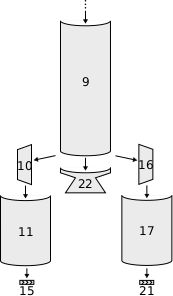
Figure 4 – A subset of the parts tree from Figure 2 above.¶
Fuel lines are considered parts, and are included in the parts tree (for example, as pictured in Figure 4). However, the parts tree does not contain information about which parts fuel lines connect to. The parent part of a fuel line is the part from which it will take fuel (as shown in Figure 4) however the part that it will send fuel to is not represented in the parts tree.
Figure 5 shows the fuel lines from the example vessel pictured earlier. Fuel line part 15 (in red) takes fuel from a fuel tank (part 11 – in green) and feeds it into another fuel tank (part 9 – in blue). The fuel line is therefore a child of part 11, but its connection to part 9 is not represented in the tree.
The attributes SpaceCenter.Part.fuel_lines_from
and
SpaceCenter.Part.fuel_lines_to
can be used to discover these
connections. In the example in Figure 5, when
SpaceCenter.Part.fuel_lines_to
is called on fuel tank part
11, it will return a list of parts containing just fuel tank part 9 (the blue
part). When SpaceCenter.Part.fuel_lines_from
is called on
fuel tank part 9, it will return a list containing fuel tank parts 11 and 17
(the parts colored green).
Staging¶
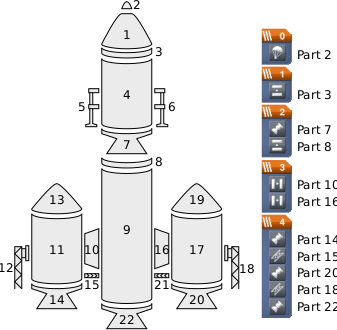
Figure 6 – Example vessel from Figure 1 with a staging sequence.¶
Each part has two staging numbers associated with it: the stage in which the
part is activated and the stage in which the part is decoupled. These values
can be obtained using SpaceCenter.Part.stage
and
SpaceCenter.Part.decouple_stage
respectively. For parts that
are not activated by staging, SpaceCenter.Part.stage
returns
-1. For parts that are never decoupled,
SpaceCenter.Part.decouple_stage
returns a value of -1.
Figure 6 shows an example staging sequence for a vessel. Figure 7 shows the stages in which each part of the vessel will be activated. Figure 8 shows the stages in which each part of the vessel will be decoupled.
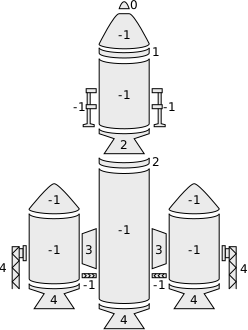
Figure 7 – The stage in which each part is activated.¶
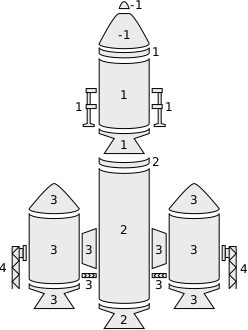
Figure 8 – The stage in which each part is decoupled.¶